2025 JS Static Methods Constructor Methods and Prototypes :
2025 JS Static Methods Constructor Methods and Prototypes – JavaScript provides different ways to create and manage objects efficiently. Static methods belong to a class rather than an object, allowing us to call them without creating an instance.
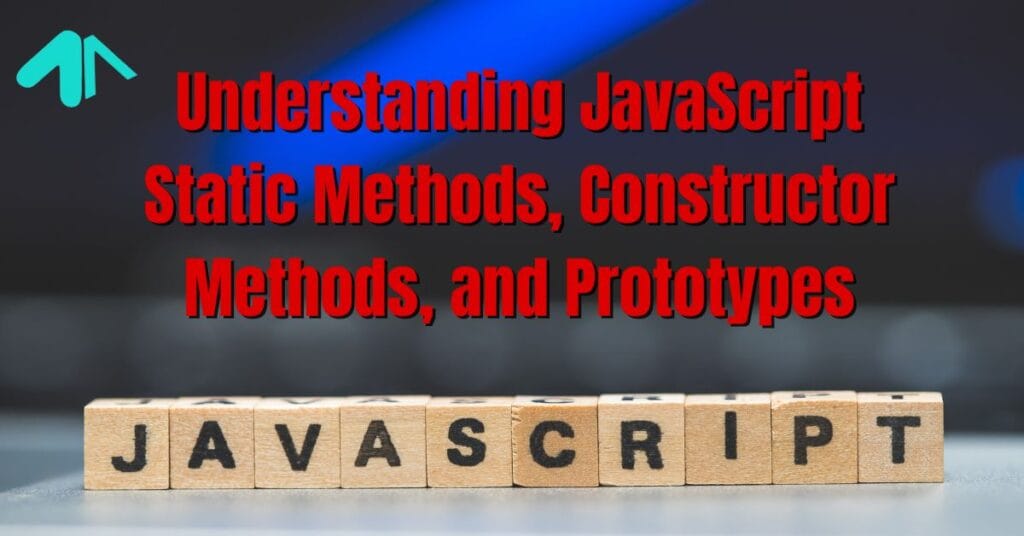
Constructor methods help in initializing new objects by assigning values when an instance is created. Prototypes allow objects to share properties and methods, improving performance and reducing memory usage.
Understanding these concepts helps developers write cleaner and more organized code, making JavaScript programs more efficient and scalable.
JavaScript Prototype: A Complete Guide in Simple Words
Introduction
JavaScript is a powerful programming language that allows developers to build interactive websites and applications. One of its key features is prototypes, which help in creating reusable and efficient code.

If you have worked with objects in JavaScript, you have already used prototypes—even if you didn’t realize it. Understanding JavaScript prototypes will help you write better and more optimized code.
What is a Prototype in JavaScript?
A prototype is like a blueprint or a shared recipe for JavaScript objects. Every object in JavaScript is linked to a prototype, which allows it to inherit properties and methods. This helps in reducing memory usage because instead of creating the same function for each object, JavaScript allows objects to share functions through prototypes.
Example:
Imagine you own a car rental service. Instead of writing separate instructions for each car, you create a standard manual (prototype) that every car follows. This saves time and ensures all cars have the same features.
Similarly, in JavaScript, objects inherit properties and methods from their prototype, just like cars follow the standard manual.
Understanding Prototype in Objects
When you create an object in JavaScript, it automatically has access to built-in properties and methods through its prototype.
Example:
let person = {
name: "Alice",
age: 25
};
console.log(person.toString()); // Output: [object Object]
Even though toString()
is not directly defined inside the person
object, it still works because it comes from the prototype.
Creating a Prototype in JavaScript
In JavaScript, every function has a special property called prototype that allows us to add methods and properties.
Example:
function Person(name, age) {
this.name = name;
this.age = age;
}
// Adding a method to the prototype
Person.prototype.greet = function() {
return `Hello, my name is ${this.name}.`;
};
let user1 = new Person("Bob", 30);
let user2 = new Person("Charlie", 28);
console.log(user1.greet()); // Output: Hello, my name is Bob.
console.log(user2.greet()); // Output: Hello, my name is Charlie.
Here, instead of adding greet()
to each object separately, we added it to the Person prototype, so all instances of Person
share this method.
Prototype Inheritance
Prototype inheritance allows one object to inherit properties and methods from another.
Example:
function Animal(name) {
this.name = name;
}
Animal.prototype.sound = function() {
return "Some generic animal sound";
};
function Dog(name, breed) {
Animal.call(this, name); // Inherit name property
this.breed = breed;
}
Dog.prototype = Object.create(Animal.prototype); // Inherit methods
Dog.prototype.constructor = Dog;
Dog.prototype.bark = function() {
return "Woof! Woof!";
};
let myDog = new Dog("Buddy", "Labrador");
console.log(myDog.name); // Output: Buddy
console.log(myDog.sound()); // Output: Some generic animal sound
console.log(myDog.bark()); // Output: Woof! Woof!
Here, Dog
inherits from Animal
, allowing it to use the sound()
method from the prototype.
Prototype vs. proto
There are two commonly used terms in JavaScript when working with prototypes:
- prototype: This is a property of constructor functions that allows us to define methods and properties that instances will inherit.
- proto: This is a property that every object has, which points to its prototype.
Example:
let obj = {};
console.log(obj.__proto__ === Object.prototype); // Output: true
Modifying Built-in Prototypes
JavaScript allows modifying built-in prototypes, but it’s not always recommended.
Example:
Array.prototype.sum = function() {
return this.reduce((acc, num) => acc + num, 0);
};
let numbers = [1, 2, 3, 4, 5];
console.log(numbers.sum()); // Output: 15
Here, we added a sum()
method to the Array
prototype, allowing all arrays to use it.
When to Use Prototypes?
- When you need multiple objects to share the same method efficiently.
- When you want to extend existing JavaScript objects.
- When working with object-oriented programming in JavaScript.
JavaScript Constructor Method: A Complete Guide
Introduction
JavaScript is widely used for building dynamic and interactive web applications. One of its key features is object-oriented programming (OOP), where we use objects to structure our code.
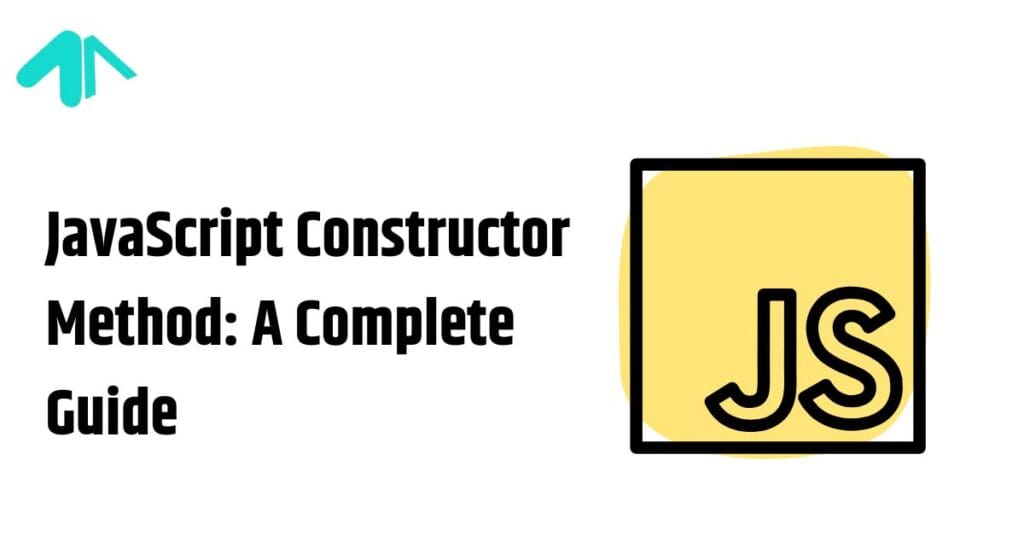
The constructor method in JavaScript is an essential part of OOP, as it helps create and initialize objects.
What is a Constructor Method in JavaScript?
A constructor method is a special function inside a class or function that initializes objects with specific properties and values. It runs automatically when a new object is created. In JavaScript, we can create constructors using constructor functions or ES6 classes.
Real-Life Example:
Think of a constructor as a blueprint for building houses. If you want to build many houses with the same structure but different colors and sizes, you create a blueprint first. Then, every time you build a house, you follow the same structure but apply different colors and sizes. In JavaScript, the constructor method works the same way to create multiple objects with similar properties.
Creating a Constructor Function
Before ES6 introduced classes, developers used constructor functions to create objects.
Example:
function Person(name, age) {
this.name = name;
this.age = age;
this.greet = function() {
return `Hello, my name is ${this.name} and I am ${this.age} years old.`;
};
}
let person1 = new Person("Alice", 25);
let person2 = new Person("Bob", 30);
console.log(person1.greet()); // Output: Hello, my name is Alice and I am 25 years old.
console.log(person2.greet()); // Output: Hello, my name is Bob and I am 30 years old.
In this example:
Person
is a constructor function.this.name
andthis.age
assign values to each new object.- The
greet
function is unique for each object created.
Creating a Constructor Using ES6 Classes
With ES6, JavaScript introduced classes, making it easier to create objects using constructors.
Example:
class Person {
constructor(name, age) {
this.name = name;
this.age = age;
}
greet() {
return `Hello, my name is ${this.name} and I am ${this.age} years old.`;
}
}
let user1 = new Person("Charlie", 28);
let user2 = new Person("David", 35);
console.log(user1.greet()); // Output: Hello, my name is Charlie and I am 28 years old.
console.log(user2.greet()); // Output: Hello, my name is David and I am 35 years old.
Here, we use the constructor
inside a class to initialize name
and age
. The greet() method is shared among all objects instead of being created separately for each object.
The Role of new
Keyword
When calling a constructor function or class, we use the new
keyword:
- It creates a new object.
- It assigns
this
to the newly created object. - It returns the object.
Without new
, the constructor function would not work as expected.
Example Without new
:
let user = Person("Eve", 40);
console.log(user); // Output: undefined (incorrect usage)
This would cause an error because new
was not used.
Adding Methods to a Prototype
Instead of defining functions inside the constructor, we can add them to the prototype.
Example:
function Car(brand, model) {
this.brand = brand;
this.model = model;
}
Car.prototype.getDetails = function() {
return `This is a ${this.brand} ${this.model}.`;
};
let car1 = new Car("Toyota", "Corolla");
let car2 = new Car("Honda", "Civic");
console.log(car1.getDetails()); // Output: This is a Toyota Corolla.
console.log(car2.getDetails()); // Output: This is a Honda Civic.
By using Car.prototype.getDetails
, we save memory by not creating separate functions for each object.
Using Default Values in Constructor
We can set default values in the constructor to prevent errors.
Example:
class Animal {
constructor(name = "Unknown", sound = "Silent") {
this.name = name;
this.sound = sound;
}
makeSound() {
return `${this.name} makes a ${this.sound} sound.`;
}
}
let cat = new Animal("Cat", "Meow");
let unknownAnimal = new Animal();
console.log(cat.makeSound()); // Output: Cat makes a Meow sound.
console.log(unknownAnimal.makeSound()); // Output: Unknown makes a Silent sound.
This prevents errors when no values are provided.
Extending a Constructor (Inheritance)
We can extend a class or constructor function to create new objects with additional features.
Example:
class Vehicle {
constructor(type, speed) {
this.type = type;
this.speed = speed;
}
move() {
return `This ${this.type} moves at ${this.speed} km/h.`;
}
}
class Bike extends Vehicle {
constructor(type, speed, brand) {
super(type, speed); // Calls the parent constructor
this.brand = brand;
}
bikeInfo() {
return `${this.brand} bike is fast!`;
}
}
let myBike = new Bike("Bike", 50, "Yamaha");
console.log(myBike.move()); // Output: This Bike moves at 50 km/h.
console.log(myBike.bikeInfo()); // Output: Yamaha bike is fast!
Here, Bike
inherits properties and methods from Vehicle
using super()
.
When to Use Constructors?
- When creating multiple objects with the same structure.
- When organizing code using Object-Oriented Programming.
- When needing default values for objects.
JavaScript Static Methods: A Complete Guide
Introduction
JavaScript provides a powerful way to create reusable code using classes and objects. Among these, static methods play an essential role in writing structured and efficient programs.
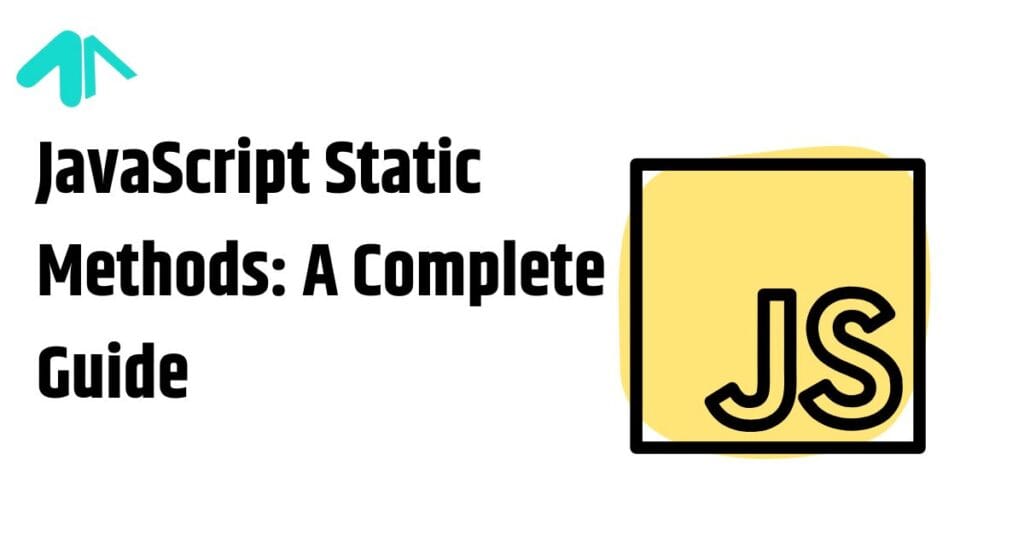
Unlike regular methods, static methods belong to a class itself rather than to instances of the class. This means they can be called directly on the class without creating an object.
What is a Static Method in JavaScript?
A static method is a method that belongs to a class instead of an object. These methods cannot be accessed from instances of the class but can be called directly on the class itself.
Example:
class MathOperations {
static add(a, b) {
return a + b;
}
}
console.log(MathOperations.add(5, 10)); // Output: 15
Here:
add()
is a static method.- It belongs to the
MathOperations
class. - It is called using
MathOperations.add()
instead of creating an instance.
Real-Life Example
Imagine you have a calculator app. Instead of creating a new calculator object every time you perform an addition, you can directly use a static method to do calculations.
Example:
class Calculator {
static multiply(a, b) {
return a * b;
}
}
console.log(Calculator.multiply(4, 3)); // Output: 12
This makes the method efficient and easily accessible.
Creating Static Methods in JavaScript Classes
To define a static method inside a class, use the static
keyword before the method name.
Example:
class Utility {
static greet(name) {
return `Hello, ${name}!`;
}
}
console.log(Utility.greet("Alice")); // Output: Hello, Alice!
greet()
is a static method.- It is called directly using
Utility.greet()
. - No instance of
Utility
is needed.
Static vs Instance Methods
Feature | Static Method | Instance Method |
---|---|---|
Called on | Class itself | Object instance |
Uses this ? | No | Yes |
Requires new ? | No | Yes |
Example | ClassName.method() | object.method() |
Example Comparison:
class Example {
static staticMethod() {
return "I am a static method";
}
instanceMethod() {
return "I am an instance method";
}
}
let obj = new Example();
console.log(Example.staticMethod()); // Works
console.log(obj.instanceMethod()); // Works
console.log(obj.staticMethod()); // Error
Trying to call a static method on an instance will throw an error.
When to Use Static Methods?
Static methods are useful when:
- You need utility functions (e.g., mathematical calculations, string manipulations).
- You want to create helper methods that don’t depend on instance properties.
- You need global behavior, such as a logging system or configuration settings.
Example: Using Static Methods for Utility Functions
Example:
class StringHelper {
static toUpperCase(str) {
return str.toUpperCase();
}
}
console.log(StringHelper.toUpperCase("hello")); // Output: HELLO
Here, toUpperCase()
is a static method that converts a string to uppercase.
Example: Using Static Methods for Counting Objects
Static methods can also track data shared across multiple instances.
Example:
class Counter {
static count = 0;
constructor() {
Counter.count++;
}
static getCount() {
return `Total instances: ${Counter.count}`;
}
}
let c1 = new Counter();
let c2 = new Counter();
console.log(Counter.getCount()); // Output: Total instances: 2
count
is a static property.getCount()
is a static method used to track the number of instances.
Using Static Methods with Inheritance
Static methods can be inherited by subclasses.
Example:
class Parent {
static showMessage() {
return "Hello from Parent class!";
}
}
class Child extends Parent {}
console.log(Child.showMessage()); // Output: Hello from Parent class!
- The
Child
class inherits theshowMessage()
method fromParent
.
Common Mistakes with Static Methods
- Trying to call a static method on an instance
let obj = new MathOperations();
console.log(obj.add(5, 5)); // Error: obj.add is not a function
Static methods should always be called on the class.
- Using
this
inside a static method
class Example {
static show() {
console.log(this);
}
}
Example.show(); // Output: [Function: Example]
this
inside a static method refers to the class itself, not an instance.
FAQs
1. What is a JavaScript static method?
A static method is a function that belongs to a class rather than an object. It can be called directly using the class name without creating an instance.
2. How do I define a static method in JavaScript?
You can define a static method using the static
keyword before the method name.
Example:class MathUtils {
static add(a, b) {
return a + b;
}
}
console.log(MathUtils.add(5, 10)); // Output: 15
3. What is a constructor method in JavaScript?
A constructor method is a special function in a class that runs when a new object is created. It helps set up object properties.
4. How do I use the constructor method?
Example:class Person { constructor(name, age) { this.name = name; this.age = age; } } let p1 = new Person("Alice", 25); console.log(p1.name); // Output: Alice
5. What is the prototype in JavaScript?
A prototype is a built-in mechanism in JavaScript that allows objects to inherit properties and methods from other objects.
6. Can static methods access instance properties?
No, static methods do not have access to instance properties because they belong to the class, not the object.
7. What is the difference between a constructor method and a prototype method?
Constructor Method: Runs when an object is created and sets up initial properties.
Prototype Method: Shared among all objects of the same type to save memory.
8. Can a static method be inherited?
Yes, static methods can be inherited by child classes.
Example:class Parent {
static greet() {
return "Hello from Parent!";
}
}
class Child extends Parent {}
console.log(Child.greet()); // Output: Hello from Parent!
Summary
JavaScript static methods, constructor methods, and prototypes are essential concepts for object-oriented programming. Static methods belong to the class and are used for utility functions. Constructor methods initialize object properties when a new object is created. Prototypes allow objects to share methods, improving memory efficiency. Understanding these concepts helps in writing clean, reusable, and scalable JavaScript code.
JavaScript Essentials: Understanding Functions, Classes, and Objects