2025 Python Control Flow and Loops
Introduction
Python is a popular programming language known for its simplicity and versatility. One of the key aspects of programming in Python is understanding control flow and loops.
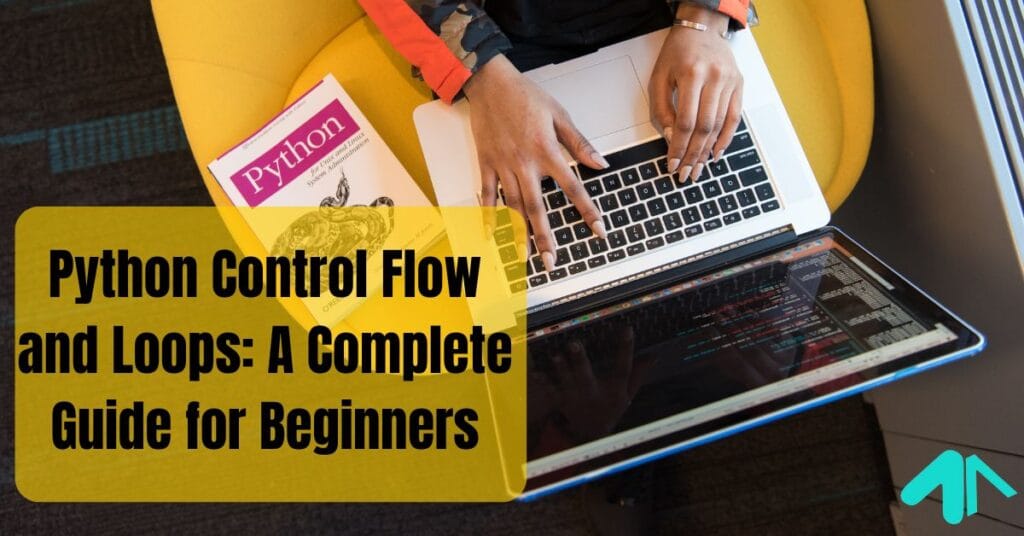
These concepts allow programmers to make decisions, repeat tasks, and control the execution of code efficiently.
What is Control Flow?
Control flow refers to the order in which statements are executed in a program. Normally, Python executes code line by line from top to bottom. However, sometimes we need to make decisions, repeat tasks, or alter the sequence of execution. This is where control flow statements come into play.
Control flow in Python includes:
- Conditional Statements (if, elif, else)
- Loops (for, while)
- Loop Control Statements (break, continue, pass)
Let’s explore each one in detail.
1. Conditional Statements
Conditional statements allow a program to make decisions based on specific conditions. In Python, we use if, elif, and else for decision-making.
1.1 The if
Statement
The if
statement checks a condition. If the condition is True
, the code inside the if
block runs.
Example:
age = 18
if age >= 18:
print("You are eligible to vote.")
Output:
You are eligible to vote.
1.2 The if-else
Statement
If the condition is False
, the code inside the else
block runs.
Example:
age = 16
if age >= 18:
print("You can vote.")
else:
print("You cannot vote.")
Output:
You cannot vote.
1.3 The if-elif-else
Statement
When there are multiple conditions, we use elif
(short for “else if”).
Example:
marks = 85
if marks >= 90:
print("Grade: A")
elif marks >= 75:
print("Grade: B")
elif marks >= 60:
print("Grade: C")
else:
print("Grade: D")
Output:
Grade: B
2. Loops in Python
Loops allow us to execute a block of code multiple times. Python has two types of loops:
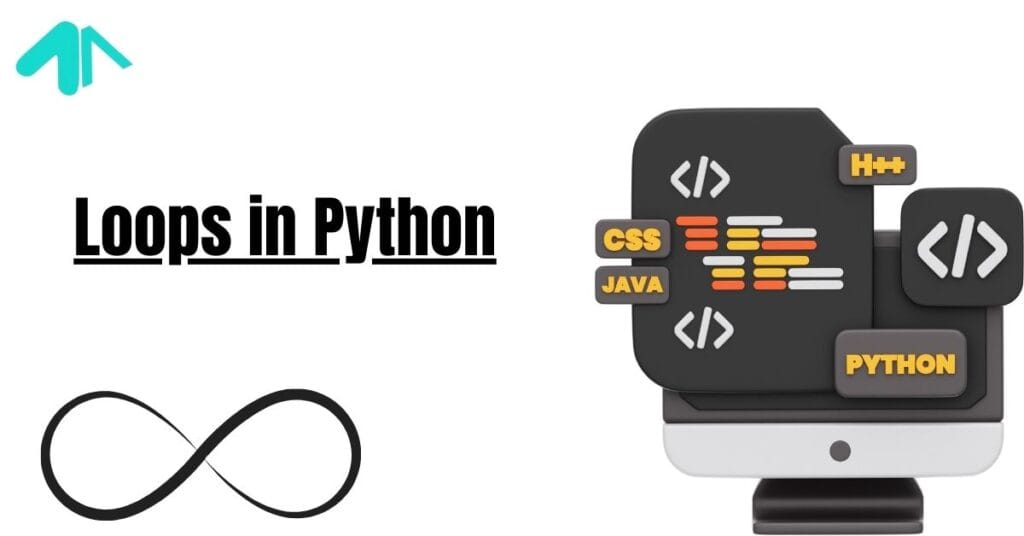
- for loop
- while loop
2.1 The for
Loop
A for
loop is used when we know how many times we want to execute a block of code. It is commonly used with lists, tuples, strings, and ranges.
Example:
for i in range(5):
print("Hello, World!")
Output:
Hello, World!
Hello, World!
Hello, World!
Hello, World!
Hello, World!
Looping Through a List:
fruits = ["apple", "banana", "cherry"]
for fruit in fruits:
print(fruit)
Output:
apple
banana
cherry
Looping Through a String:
word = "Python"
for letter in word:
print(letter)
Output:
P
y
t
h
o
n
2.2 The while
Loop
A while
loop runs as long as the condition is True
.
Example:
count = 1
while count <= 5:
print(count)
count += 1
Output:
1
2
3
4
5
Be careful with while
loops! If the condition never becomes False
, the loop will run forever (infinite loop).
Example of an Infinite Loop:
while True:
print("This is an infinite loop!")
(This will keep running unless manually stopped.)
3. Loop Control Statements
Loop control statements help us control the execution of loops. Python has three such statements:
3.1 The break
Statement
The break
statement stops the loop immediately.
Example:
for number in range(10):
if number == 5:
break
print(number)
Output:
0
1
2
3
4
3.2 The continue
Statement
The continue
statement skips the rest of the code in the loop for the current iteration and moves to the next iteration.
Example:
for number in range(5):
if number == 2:
continue
print(number)
Output:
0
1
3
4
3.3 The pass
Statement
The pass
statement does nothing. It is used as a placeholder when a statement is required but no action needs to be taken.
Example:
for number in range(5):
if number == 3:
pass
print(number)
Output:
0
1
2
3
4
4. Nested Loops
A nested loop means having one loop inside another loop.
Example:
for i in range(3):
for j in range(3):
print(f"i={i}, j={j}")
Output:
i=0, j=0
i=0, j=1
i=0, j=2
i=1, j=0
i=1, j=1
i=1, j=2
i=2, j=0
i=2, j=1
i=2, j=2
FAQs – 2025 Python Control Flow and Loops
1. What is the difference between break
and continue
statements?
Break exits the loop completely, while continue skips the current iteration and proceeds with the next one.
2. Can we use else
with loops in Python?
Yes, Python allows using else
with loops. The else
block runs only if the loop completes without encountering a break
statement.for i in range(5):
print(i)
else:
print("Loop finished successfully")
3. How does Python handle infinite loops?
An infinite loop occurs when the condition in a while
loop never becomes False
. To stop execution, press Ctrl + C.while True:
print("This is an infinite loop")
4. What is the best loop to use when iterating over a list?
A for
loop is generally more efficient and readable when iterating over a list.for item in my_list: print(item)
I hope this guide helps you understand Python’s control flow and loops in detail! Keep practicing and experimenting with different use cases.
Summary
In this article, we explored Python’s control flow mechanisms and looping constructs in depth. We learned about Boolean logic, conditional statements (if
, if-else
, if-elif-else
), and loops (while
, for
). Additionally, we examined practical applications of these concepts to solve real-world problems.
Understanding Python: Flow of Execution and Parameters & Arguments