Voting Contract App: Preparing for Smart Contract Development
Introduction
Case Study for Blockchain 2025 – Voting is an essential part of decision-making in various aspects of life, from political elections to community decisions and corporate governance. Traditional voting methods often face challenges such as fraud, lack of transparency, and inefficiency. With blockchain technology, these problems can be minimized using smart contracts. A Voting Contract App ensures security, transparency, and automation in elections.
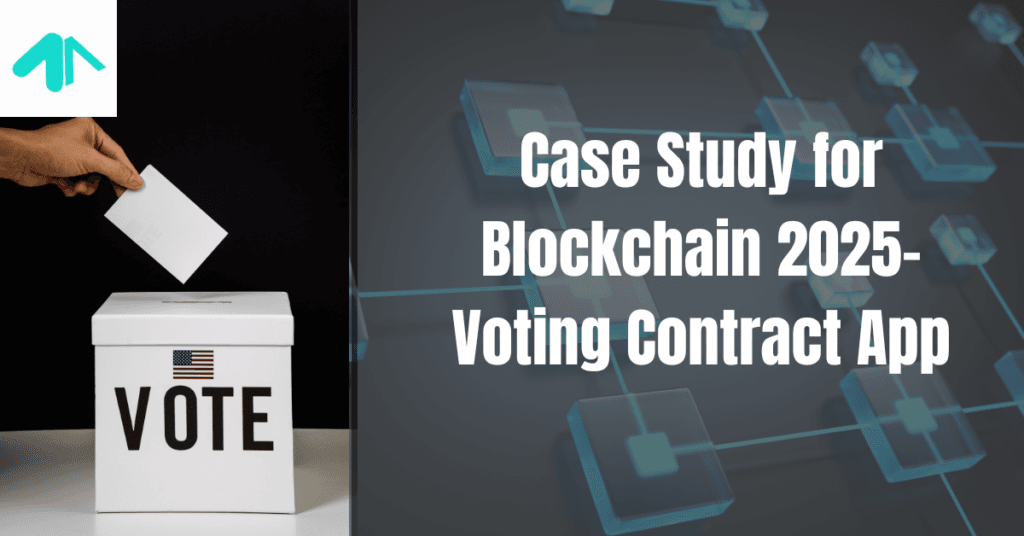
If you are planning to develop a voting contract app, you need to understand smart contract development, blockchain platforms, and Ethereum frameworks.
What is a Smart Contract?
A smart contract is a self-executing contract with predefined rules written in code. It operates on a blockchain network, ensuring security and transparency without intermediaries. In a voting contract app, a smart contract ensures:
- Only eligible voters can participate.
- Votes are recorded immutably on the blockchain.
- The voting process is automated and tamper-proof.
Why Use Blockchain for Voting?
Using blockchain technology in voting systems offers several benefits:
- Transparency – All votes are recorded on the blockchain, making the process transparent.
- Security – Blockchain’s cryptographic nature ensures vote integrity.
- Elimination of Fraud – Prevents duplicate voting and tampering.
- Automation – The process is executed without manual intervention.
- Anonymity – Ensures voters’ privacy.
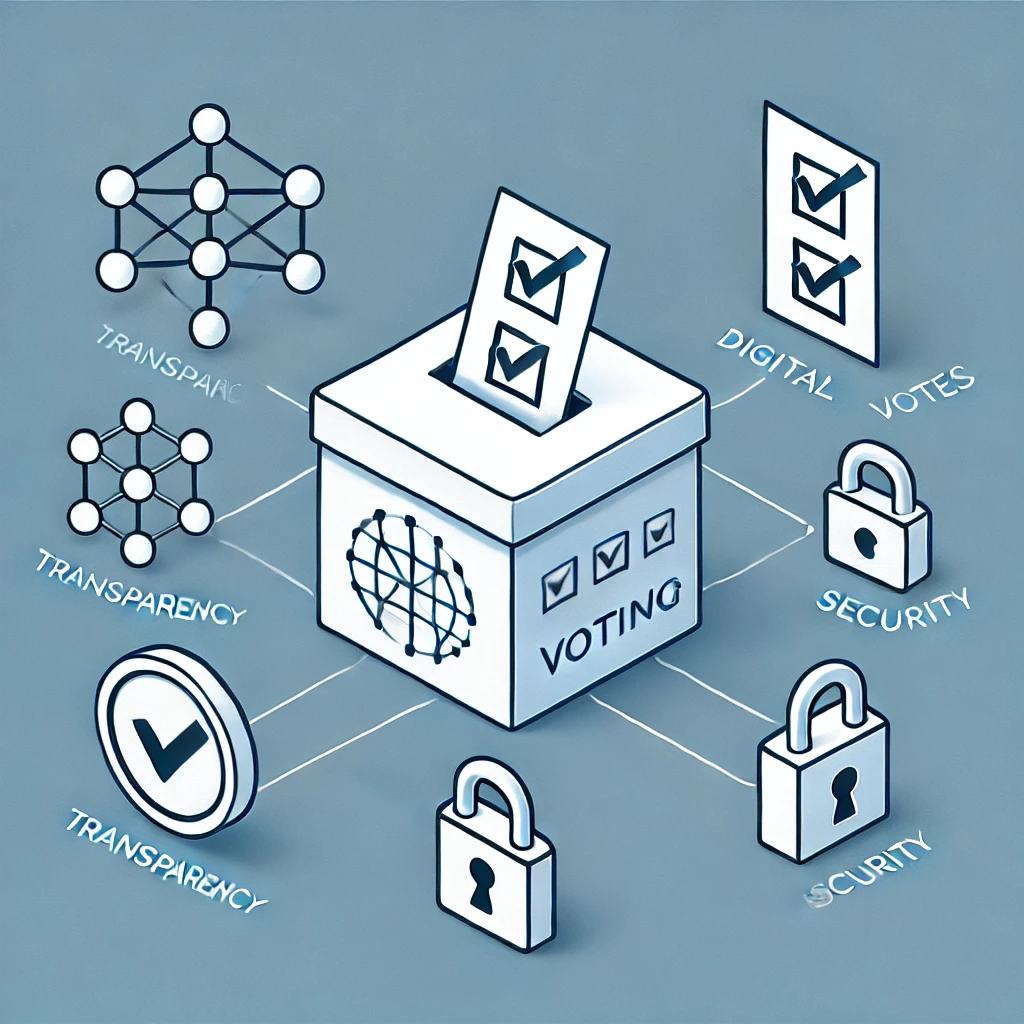
Steps to Develop a Voting Contract App
1. Define the Requirements
Before writing a smart contract, outline the requirements:
- Who can vote? (Voter eligibility criteria)
- How will voters be authenticated?
- What voting system will be used (simple majority, ranked voting, etc.)?
- How will votes be counted and displayed?
2. Choose the Blockchain Platform
Ethereum is the most popular blockchain for smart contracts. You can use Ethereum-based tools like:
- Remix IDE – A browser-based Solidity compiler.
- Ganache – A local Ethereum blockchain for testing.
- Metamask – A wallet for interacting with Ethereum networks.
- Etherscan – A blockchain explorer for verifying transactions.
3. Set Up the Development Environment
To start coding your smart contract, install:
npm install -g truffle
npm install -g ganache-cli
This will set up Truffle (a smart contract development framework) and Ganache (a local test blockchain).
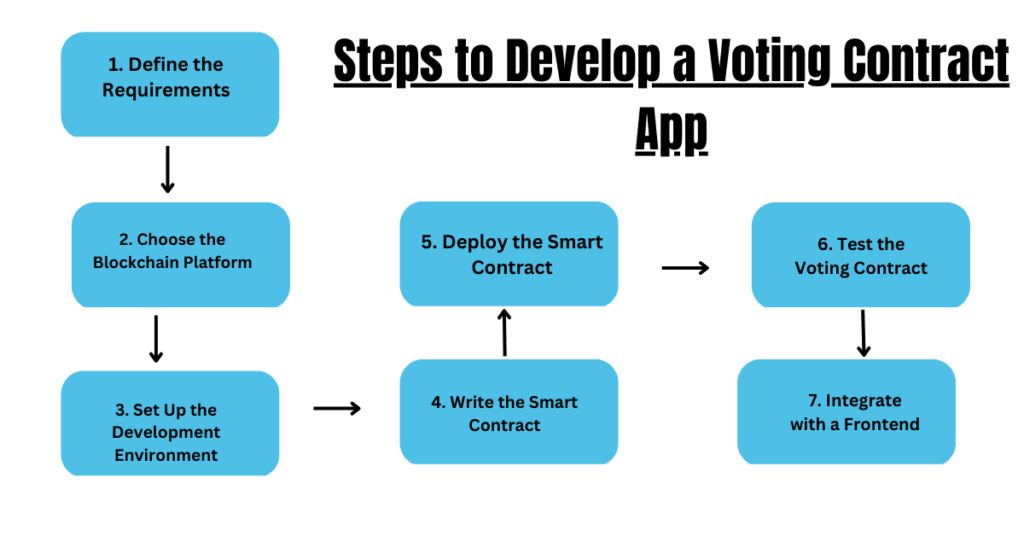
4. Write the Smart Contract
Here’s a basic Solidity smart contract for voting:
pragma solidity ^0.8.0;
contract Voting {
struct Candidate {
string name;
uint voteCount;
}
mapping(address => bool) public hasVoted;
Candidate[] public candidates;
constructor(string[] memory candidateNames) {
for (uint i = 0; i < candidateNames.length; i++) {
candidates.push(Candidate({name: candidateNames[i], voteCount: 0}));
}
}
function vote(uint candidateIndex) public {
require(!hasVoted[msg.sender], "You have already voted.");
candidates[candidateIndex].voteCount++;
hasVoted[msg.sender] = true;
}
function getResults() public view returns (string memory winner) {
uint winningVoteCount = 0;
for (uint i = 0; i < candidates.length; i++) {
if (candidates[i].voteCount > winningVoteCount) {
winningVoteCount = candidates[i].voteCount;
winner = candidates[i].name;
}
}
}
}
5. Deploy the Smart Contract
Use Truffle or Remix IDE to deploy the contract:
truffle migrate --network development
This will deploy the contract on the test network.
6. Test the Voting Contract
Testing ensures the contract functions correctly. You can write test cases using JavaScript and the Truffle testing framework:
const Voting = artifacts.require("Voting");
contract("Voting", (accounts) => {
it("should allow a voter to vote", async () => {
let instance = await Voting.deployed();
await instance.vote(0, {from: accounts[0]});
let candidate = await instance.candidates(0);
assert(candidate.voteCount.toNumber() === 1, "Vote not recorded");
});
});
Run the test using:
truffle test
7. Integrate with a Frontend
To make the voting contract user-friendly, create a simple frontend using React.js:
- Web3.js or ethers.js for blockchain interaction.
- Metamask for authentication.
- A UI to display candidates and results.
8. Deploy on Ethereum Mainnet
Once tested, deploy your smart contract on the Ethereum Mainnet or Polygon (Layer 2) using:
truffle migrate --network mainnet
Make sure to have ETH for gas fees.
Challenges in Smart Contract Voting
- Gas Fees – High transaction costs on Ethereum.
- Scalability – Handling large-scale elections.
- Security – Protecting against hacking attempts.
- User Adoption – Educating users on blockchain voting.
Case Study for Blockchain 2025 – FAQs
1. Can a voting smart contract be hacked?
A well-written smart contract is highly secure, but vulnerabilities can exist. Regular auditing and best coding practices help prevent exploits.
2. How do voters verify their votes?
Voters can check the blockchain ledger to verify their vote’s existence without revealing their identity.
3. What happens if a voter tries to vote multiple times?
The smart contract prevents double voting by maintaining a mapping of addresses that have already voted.
4. What if there is a bug in the contract?
Once deployed on Ethereum, smart contracts cannot be modified. Testing and auditing before deployment are crucial.
5. Can smart contract voting be used for government elections?
Yes, but it requires legal approval and infrastructure readiness. Several countries are experimenting with blockchain voting solutions.
Summary
A Voting Contract App can revolutionize the way elections are conducted by ensuring security, transparency, and efficiency. By following the right steps—defining requirements, choosing Ethereum, writing smart contracts, testing, and integrating with a frontend—you can build a blockchain-powered voting system. Smart contract development requires an understanding of Solidity, blockchain frameworks, and security considerations.
Understanding Enterprise Blockchain 2025
What Are Avalanches 3 Blockchains?
Hey everyone! If you have any questions or thoughts, feel free to drop a comment. Let’s learn and discuss together. Your feedback is always welcome!