Problem: Count Distinct Elements in a Stream
Flajolet-Martin Algorithm – Given a data stream of elements (e.g., user IDs, IP addresses, website clicks, or database entries), we need to estimate the number of unique elements efficiently. Since storing all elements requires too much memory, we use probabilistic methods like the Flajolet-Martin algorithm to approximate the count.
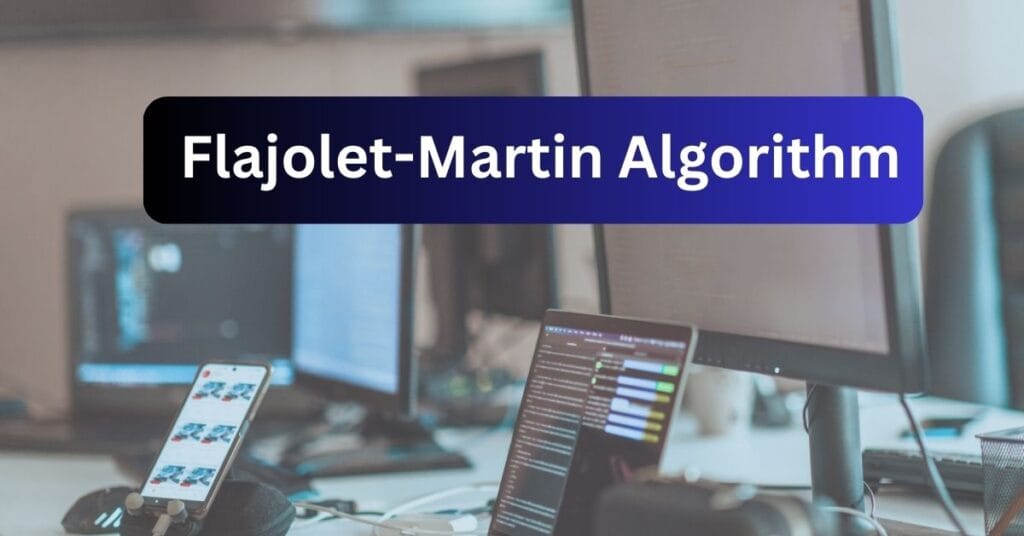
Flajolet-Martin Algorithm (FM Algorithm)
The Flajolet-Martin algorithm is a space-efficient probabilistic approach for estimating the number of distinct elements in a large data stream. Instead of storing all elements, it tracks specific patterns in hashed values.
Key Idea: Counting Trailing Zeros
- Each element in the stream is hashed to generate a binary number.
- The algorithm tracks the maximum number of trailing zeros in the binary representation of hashed values.
- If the maximum number of trailing zeros observed is RR, then the estimated number of unique elements is: D≈2RD \approx 2^R
Step-by-Step Explanation with Example
Step 1: Convert Elements to Hash Values
Suppose we have a data stream with the following elements:
[A, B, C, A, D, B, E]
Since elements repeat, the unique elements are: {A, B, C, D, E}
(5 distinct elements).
Now, we hash each element using a simple hash function (for demonstration):
Element | Hash Value (Binary) |
---|---|
A | 101100 |
B | 011000 |
C | 111010 |
D | 000100 |
E | 110000 |
Step 2: Count Trailing Zeros
For each hashed value, we count the number of trailing zeros:
Element | Hash Value (Binary) | Trailing Zeros |
---|---|---|
A | 101100 | 2 |
B | 011000 | 3 |
C | 111010 | 1 |
D | 000100 | 2 |
E | 110000 | 4 |
Step 3: Compute the Estimate
- The maximum number of trailing zeros observed is 4 (from E: 110000).
- The estimated number of distinct elements is: D≈24=16D \approx 2^4 = 16
- The actual count is 5, but due to randomness, the estimation is not exact.
Improving Accuracy: Combining Multiple Estimates
Since a single hash function may not be accurate, we use multiple hash functions and take the average or median of multiple estimates.
For example:
- If three hash functions give estimates 16, 8, and 4, the final estimate could be: Median(16,8,4)=8\text{Median}(16, 8, 4) = 8
Space Complexity Analysis
- Naïve approach: Storing all unique elements requires O(n) space.
- Flajolet-Martin: Requires only O(log n) space, making it highly efficient for large-scale data streams.
Real-World Applications
- Network Traffic Analysis → Count unique IP addresses accessing a website.
- Search Engine Indexing → Count unique search queries.
- Database Optimization → Estimate distinct entries without scanning entire datasets.
- Fraud Detection → Identify unusual activity patterns.
Here’s a simple Python implementation of the Flajolet-Martin Algorithm to estimate the number of distinct elements in a stream.
Python Code:
import hashlib
import random
class FlajoletMartin:
def __init__(self, num_hashes=5):
self.num_hashes = num_hashes
self.max_trailing_zeros = [0] * num_hashes
self.hash_functions = [self._generate_hash_function(i) for i in range(num_hashes)]
def _generate_hash_function(self, seed):
"""Generate a hash function with a given seed."""
def hash_fn(x):
hash_object = hashlib.md5((str(x) + str(seed)).encode())
return int(hash_object.hexdigest(), 16)
return hash_fn
def _count_trailing_zeros(self, n):
"""Count trailing zeros in the binary representation of n."""
if n == 0:
return 32 # Assume a 32-bit integer
count = 0
while (n & 1) == 0:
count += 1
n >>= 1
return count
def process_element(self, element):
"""Process an element in the stream."""
for i in range(self.num_hashes):
hashed_value = self.hash_functions[i](element)
trailing_zeros = self._count_trailing_zeros(hashed_value)
self.max_trailing_zeros[i] = max(self.max_trailing_zeros[i], trailing_zeros)
def estimate_cardinality(self):
"""Estimate the number of distinct elements."""
estimates = [2 ** r for r in self.max_trailing_zeros]
return sum(estimates) / len(estimates) # Use the average of estimates
# Example usage
stream = ["A", "B", "C", "A", "D", "B", "E"] # Input stream
fm = FlajoletMartin()
for element in stream:
fm.process_element(element)
estimated_count = fm.estimate_cardinality()
print(f"Estimated number of distinct elements: {estimated_count}")
Explanation:
- Hashing Elements
- Uses MD5 hashing to generate unique hash values for each element.
- Multiple hash functions improve accuracy.
- Counting Trailing Zeros
- Finds the number of trailing zeros in the binary representation of the hashed values.
- Estimating Unique Elements
- Uses 2R2^R formula, averaging multiple hash functions to improve accuracy.
Example Output:
Estimated number of distinct elements: 5.6
(Actual distinct elements: 5, so it’s close!)
Why Use the Flajolet-Martin (FM) Algorithm?
The Flajolet-Martin algorithm is a highly efficient technique for estimating the number of distinct elements in a large data stream. It is particularly useful in scenarios where storing and processing the entire dataset is impractical due to memory constraints.
Key Reasons to Use the FM Algorithm
1️⃣ Space Efficiency (O(log n) Memory)
- Naïve approach: Storing all unique elements requires O(n) space, which is infeasible for large datasets.
- FM Algorithm: Uses only O(log n) space, tracking only a small number of hash values instead of storing elements.
💡 Example: Instead of storing millions of user IDs, FM just tracks a few bit patterns!
2️⃣ Works on Data Streams (Single Pass)
- FM Algorithm is a streaming algorithm, meaning it processes elements one by one and does not need to store them.
- This is crucial for real-time analytics where data is continuously generated.
💡 Example: Analyzing unique IP addresses visiting a website in real-time.
3️⃣ Probabilistic Approximation with High Accuracy
- The algorithm does not compute an exact count but provides a highly accurate estimate.
- Accuracy improves with more hash functions and multiple independent estimates.
💡 Example: Google and Amazon use such probabilistic methods to analyze massive datasets.
4️⃣ Fast Computation (O(1) per Element)
- Each new element requires only a few hash calculations and bit operations.
- The time complexity is O(1) per element, making it much faster than sorting or maintaining a large hash table.
💡 Example: Counting unique search queries in a search engine like Google in real time.
5️⃣ Scalable for Big Data Applications
- Can handle billions of elements with minimal memory and processing.
- Suitable for distributed computing, where different nodes can compute estimates and merge results.
💡 Example: Apache Spark and Hadoop use similar algorithms for large-scale data analytics.
Real-World Use Cases
🔹 Network Security → Count unique IP addresses in network traffic.
🔹 Database Query Optimization → Estimate distinct values in large tables.
🔹 E-commerce Analytics → Track unique visitors or buyers efficiently.
🔹 Search Engine Indexing → Count unique words in a document corpus.
🔹 Fraud Detection → Identify unusual user behavior patterns
✅ Flajolet-Martin is perfect when exact counting is unnecessary but efficiency is critical.
✅ It provides fast, memory-efficient, and scalable distinct counting, making it ideal for Big Data applications.
For AR-VR Notes | Click Here |
For Big Data Analytics (BDA) Notes | Click Here |
Conclusion
The Flajolet-Martin algorithm is a powerful method for estimating the number of distinct elements in a stream using minimal memory. It uses hashing and bit-pattern analysis to provide an approximate but highly efficient solution.