HTML Tables 2025 – Tables in HTML help organize data neatly. Just like how we use tables in notebooks to list items, prices, and schedules, HTML tables arrange content systematically on web pages. In this blog, we’ll explore everything about HTML tables, from basic structure to advanced features, with easy examples and explanations.
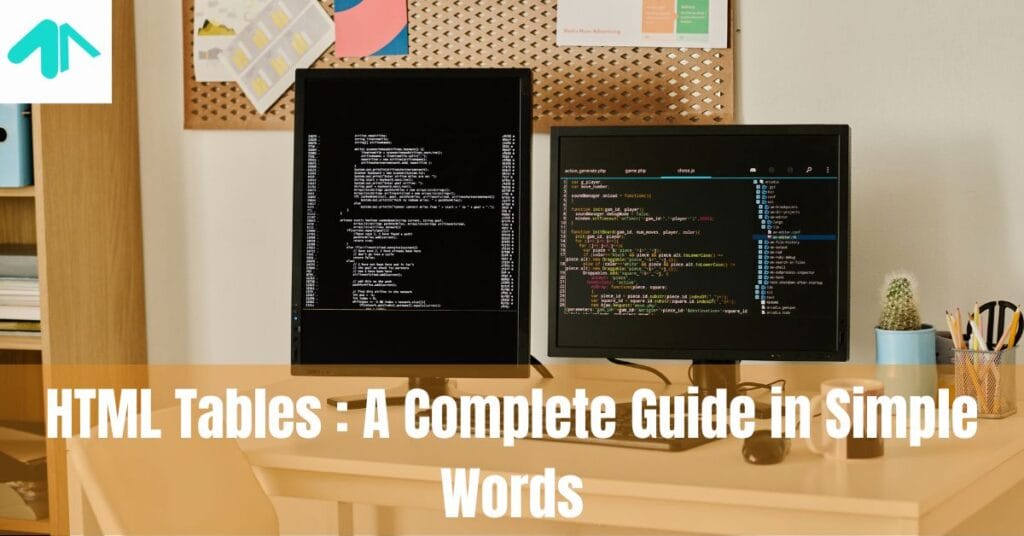
What is an HTML Table?
An HTML table is a way to display data in rows and columns. Each table consists of the following elements:
<table>
: Defines the table.<tr>
: Defines a table row.<td>
: Defines a table cell (column inside a row).<th>
: Defines a table header.
Example:
<table border="1">
<tr>
<th>Name</th>
<th>Age</th>
<th>City</th>
</tr>
<tr>
<td>John</td>
<td>25</td>
<td>New York</td>
</tr>
<tr>
<td>Emma</td>
<td>30</td>
<td>London</td>
</tr>
</table>
This creates a simple table with three columns and two rows of data.
Why Use Tables in HTML?
Tables are useful for:
- Displaying structured data (like product lists, student scores, or schedules).
- Organizing information in a clear and readable format.
- Creating comparison charts.
Adding a Table Border
By default, tables don’t have borders. You can add them using the border
attribute or CSS.
Example:
<table style="border: 2px solid black; border-collapse: collapse;">
<tr>
<th style="border: 1px solid black;">Product</th>
<th style="border: 1px solid black;">Price</th>
</tr>
<tr>
<td style="border: 1px solid black;">Laptop</td>
<td style="border: 1px solid black;">$1000</td>
</tr>
</table>
This ensures that every cell has a visible border.
Merging Cells (Colspan & Rowspan)
Sometimes, you may want to merge multiple columns or rows.
Merging Columns (colspan
):
<table border="1">
<tr>
<th colspan="2">Full Name</th>
</tr>
<tr>
<td>John Doe</td>
<td>Emma Watson</td>
</tr>
</table>
This makes the “Full Name” header span across two columns.
Merging Rows (rowspan
):
<table border="1">
<tr>
<th>Name</th>
<th>Age</th>
</tr>
<tr>
<td rowspan="2">John</td>
<td>25</td>
</tr>
<tr>
<td>26</td>
</tr>
</table>
Here, “John” spans two rows.
Styling Tables with CSS
To make tables more attractive, use CSS.
Example:
<style>
table {
width: 100%;
border-collapse: collapse;
}
th, td {
border: 1px solid black;
padding: 10px;
text-align: left;
}
th {
background-color: lightgray;
}
</style>
This adds spacing, alignment, and background color to the headers.
Adding a Caption
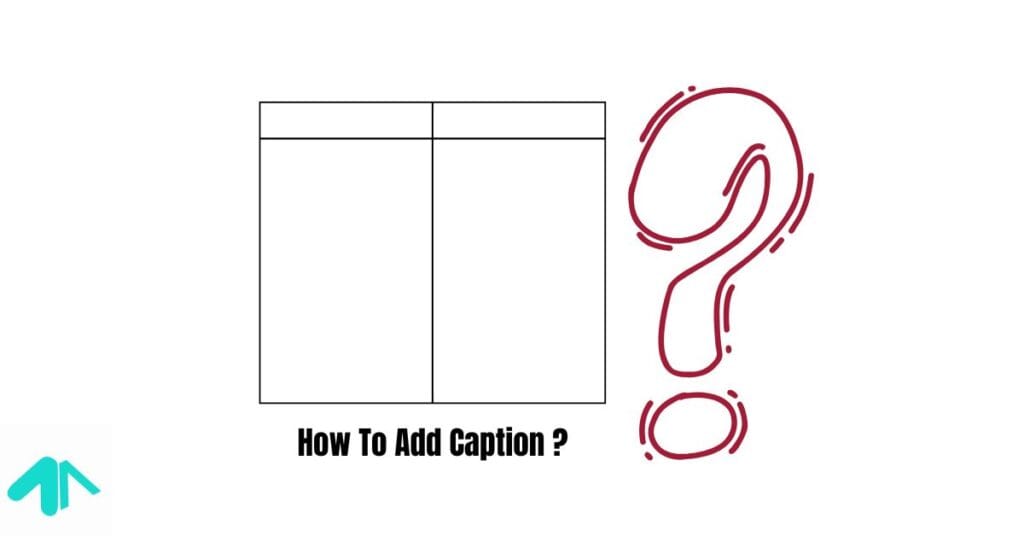
A caption describes the table’s content.
<table border="1">
<caption>Student Grades</caption>
<tr>
<th>Name</th>
<th>Grade</th>
</tr>
<tr>
<td>Alice</td>
<td>A</td>
</tr>
</table>
Responsive Tables
On smaller screens, tables should be scrollable.
<div style="overflow-x:auto;">
<table border="1">
<tr>
<th>Product</th>
<th>Price</th>
</tr>
<tr>
<td>Phone</td>
<td>$700</td>
</tr>
</table>
</div>
This ensures the table remains scrollable on mobile devices.
How to Add Borders to an HTML Table?
By default, HTML tables have no borders. You can add borders using the border
attribute or CSS.
Using the border
Attribute (Old Method)
<table border="1">
<tr>
<th>Name</th>
<th>Age</th>
</tr>
<tr>
<td>John</td>
<td>25</td>
</tr>
</table>
Using CSS (Modern Method)
<style>
table, th, td {
border: 1px solid black;
border-collapse: collapse;
}
th, td {
padding: 10px;
}
</style>
How to Add a Blank Row in an HTML Table?
A blank row can be added by inserting an empty <tr>
tag:
<table border="1">
<tr>
<td>Apple</td>
<td>Fruit</td>
</tr>
<tr>
<td colspan="2"></td>
</tr>
<tr>
<td>Carrot</td>
<td>Vegetable</td>
</tr>
</table>
How to Add Buttons Inside a Table?
<table border="1">
<tr>
<th>Name</th>
<th>Action</th>
</tr>
<tr>
<td>John</td>
<td><button>Click Me</button></td>
</tr>
</table>
How to Add a Caption to an HTML Table?
A caption describes the purpose of the table.
<table border="1">
<caption>Student Information</caption>
<tr>
<th>Name</th>
<th>Age</th>
</tr>
</table>
How to Add Background Color to Table Cells?
You can use CSS or the bgcolor
attribute.
<td style="background-color: yellow;">Text</td>
How to Add an Image Inside a Table?
<table border="1">
<tr>
<td><img src="image.jpg" width="50"></td>
<td>Product Name</td>
</tr>
</table>
How to Add a Drop-down List Inside a Table?
<table border="1">
<tr>
<td>
<select>
<option>Option 1</option>
<option>Option 2</option>
</select>
</td>
</tr>
</table>
How to Make a Scrollable Table?
<div style="overflow-x:auto;">
<table border="1">
<tr>
<th>Product</th>
<th>Price</th>
</tr>
<tr>
<td>Phone</td>
<td>$700</td>
</tr>
</table>
</div>
How to Center an HTML Table?
<style>
table {
margin: auto;
}
</style>
How to Display a SQL Table in HTML?
Use a backend language like PHP to fetch and display data from SQL.
<?php
$conn = new mysqli("localhost", "username", "password", "database");
$sql = "SELECT * FROM users";
$result = $conn->query($sql);
if ($result->num_rows > 0) {
echo "<table border='1'><tr><th>ID</th><th>Name</th></tr>";
while($row = $result->fetch_assoc()) {
echo "<tr><td>" . $row["id"] . "</td><td>" . $row["name"] . "</td></tr>";
}
echo "</table>";
} else {
echo "No results";
}
$conn->close();
?>
What is the Table Element in HTML?
The <table>
tag is used to create a table in HTML. It helps organize data in rows and columns.
Example:
<table>
<tr>
<td>Apple</td>
<td>Banana</td>
</tr>
<tr>
<td>Orange</td>
<td>Grapes</td>
</tr>
</table>
What is the Table Footer in HTML?
The <tfoot>
tag is used to define the footer section of a table, usually containing totals or summary data.
Example:
<table border="1">
<thead>
<tr>
<th>Item</th>
<th>Price</th>
</tr>
</thead>
<tbody>
<tr>
<td>Apple</td>
<td>$1</td>
</tr>
<tr>
<td>Banana</td>
<td>$2</td>
</tr>
</tbody>
<tfoot>
<tr>
<td>Total</td>
<td>$3</td>
</tr>
</tfoot>
</table>
How to Set Table Height and Width in HTML?
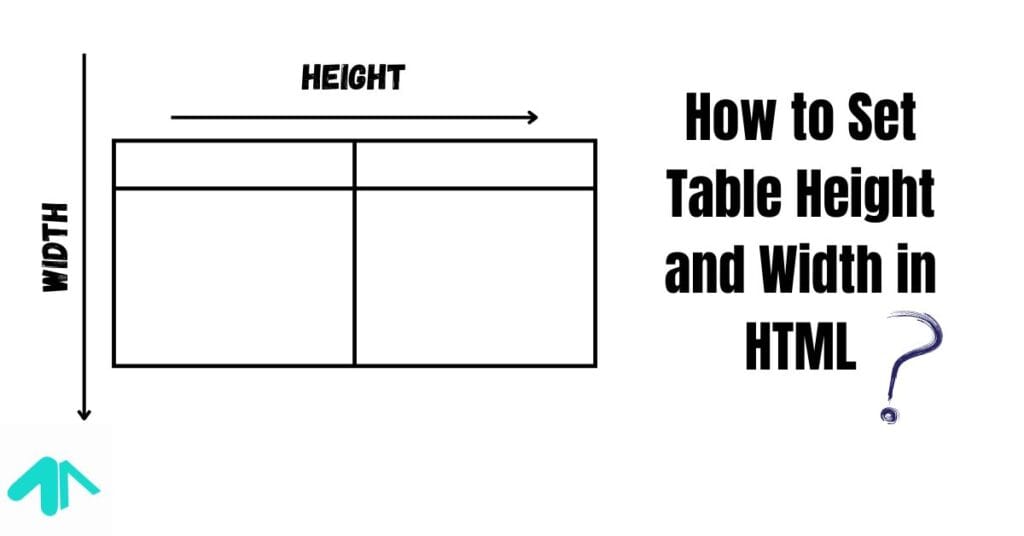
You can set the height and width using the width
and height
attributes or CSS styles.
Example:
<table style="width: 50%; height: 200px; border: 1px solid black;">
<tr>
<td>Cell 1</td>
<td>Cell 2</td>
</tr>
</table>
What is the Tag for Table Column in HTML?
The <col>
and <colgroup>
tags are used to define and style table columns.
Example:
<table border="1">
<colgroup>
<col style="background-color: lightgray;">
<col style="background-color: lightblue;">
</colgroup>
<tr>
<td>Column 1</td>
<td>Column 2</td>
</tr>
</table>
Why Use Tables in HTML?
Tables are useful for displaying data like schedules, financial reports, and structured information in a grid format.
What Replaces Tables in HTML?
For webpage layouts, use CSS Grid or Flexbox instead of tables.
Example using Flexbox:
<div style="display: flex;">
<div style="width: 50%; background-color: lightgray;">Item 1</div>
<div style="width: 50%; background-color: lightblue;">Item 2</div>
</div>
What Should Tables Not Be Used For?
Tables should not be used for webpage layouts. Instead, use CSS Grid or Flexbox for better design and responsiveness.
Alternative to <br>
in Tables
Instead of using <br>
, you can use CSS padding and margins for spacing inside table cells.
Example:
<td style="padding: 10px;">Data</td>
What Tag Defines a Standard Cell in an HTML Table?
The <td>
tag defines a standard data cell in an HTML table.
Example:
<tr>
<td>Cell 1</td>
<td>Cell 2</td>
</tr>
What to Put in an HTML Table?
Tables can contain text, numbers, images, links, and even forms.
Example:
<table border="1">
<tr>
<td><img src="image.jpg" alt="Image"></td>
<td><a href="#">Click Here</a></td>
</tr>
</table>
What to Use Instead of Tables in HTML?
If you are designing a layout, use CSS Grid or Flexbox instead of tables for better responsiveness.
Example using CSS Grid:
<div style="display: grid; grid-template-columns: 1fr 1fr;">
<div style="background-color: lightgray;">Item 1</div>
<div style="background-color: lightblue;">Item 2</div>
</div>
HTML Tables 2025 – FAQs
1. Can I add images inside a table?
Yes, simply use the <img>
tag inside a <td>
.<td><img src="phone.jpg" width="50"></td>
2. How do I center text inside a table cell?
Use CSS:<td style="text-align: center;">Centered Text</td>
3. How do I make my table have even column widths?
Use CSS:table { width: 100%; }
th, td { width: 50%; }
4. How do I add alternating row colors?
Use:tr:nth-child(even) { background-color: #f2f2f2; }
Summary
Tables in HTML help organize and display data effectively. With proper styling and attributes, tables become more readable and visually appealing. Try experimenting with tables in your next web project!
Now that you know how to use HTML tables, start practicing and enhance your web design skills!