Introduction
JavaScript 2025 – If you’ve ever wondered how websites come to life with interactive buttons, animations, and pop-ups, the answer is JavaScript. JavaScript (JS) is a programming language that makes web pages dynamic and engaging. Unlike HTML and CSS, which define the structure and design of a page, JavaScript adds behavior, allowing users to interact with the site.
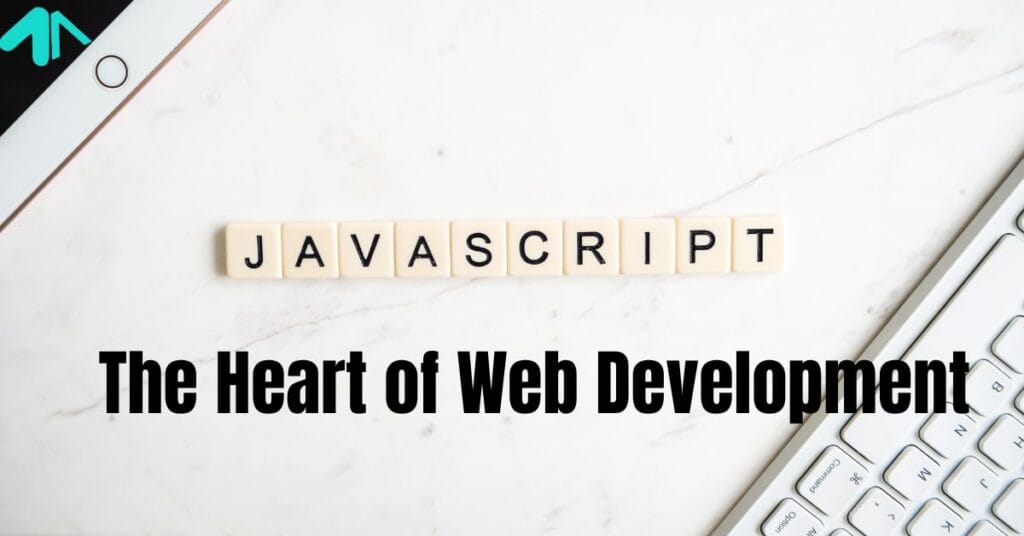
What is JavaScript?
JavaScript is a scripting language used to create and control interactive elements on websites. It works with HTML and CSS to provide a complete user experience.
Think of a website as a human body:
- HTML is the skeleton, giving the site structure.
- CSS is the clothing, making it look attractive.
- JavaScript is the brain, making decisions and responding to user actions.
For example, when you click a button and a pop-up appears, JavaScript is at work.
How JavaScript Works in a Website
When you visit a website, your browser reads its code, including JavaScript, and executes it. JavaScript runs directly in the browser, meaning you don’t need extra software to see its effects.
You can add JavaScript to a webpage in three ways:
- Inline JavaScript – Directly inside an HTML tag.
<button onclick="alert('Hello!')">Click me</button>
- Internal JavaScript – Inside a
<script>
tag within the HTML file.<script> function sayHello() { alert("Hello!"); } </script>
- External JavaScript – In a separate
.js
file and linked to the HTML file.<script src="script.js"></script>
Basic JavaScript Concepts
To understand JavaScript, let’s explore its basic concepts using everyday examples.
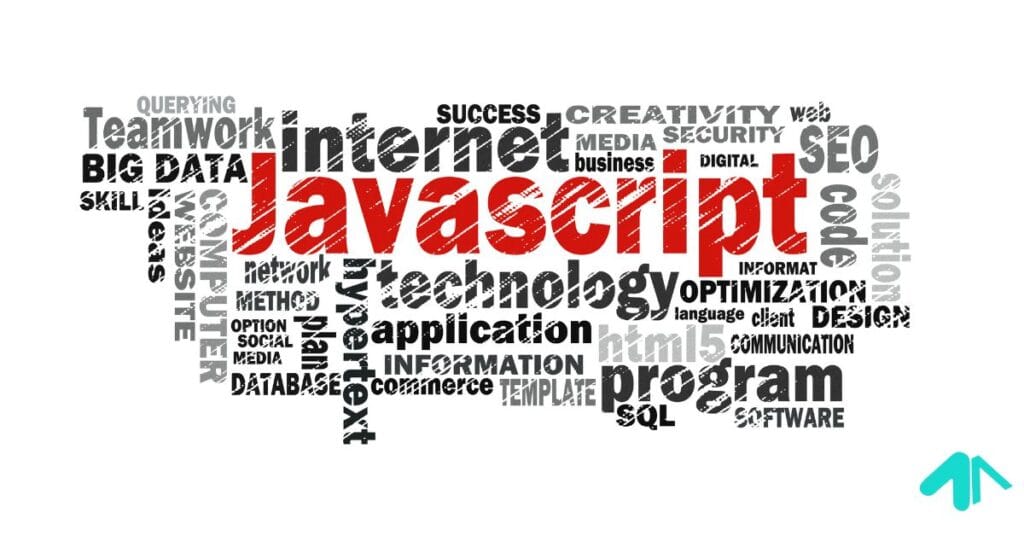
1. Variables: Storing Information
Variables are like boxes where you store data. For example:
var name = "John";
let age = 25;
const city = "New York";
var
,let
, andconst
create variables.name
stores “John” (a string).age
stores 25 (a number).city
stores “New York” (a string).
Think of a variable as a label on a storage box. You put something inside and can retrieve it anytime.
2. Functions: Reusable Code
A function is a block of code that does something useful.
function greet() {
console.log("Hello, welcome to JavaScript!");
}
When you call greet();
, the function runs and prints the message.
Think of a function as a coffee machine: once you press a button, it makes coffee for you.
3. Conditional Statements: Making Decisions
JavaScript can make decisions based on conditions.
let time = 18;
if (time < 12) {
console.log("Good morning!");
} else {
console.log("Good evening!");
}
This is like deciding whether to take an umbrella: if it’s raining, you take one; if not, you don’t.
4. Loops: Repeating Tasks
Loops help repeat tasks without writing the same code multiple times.
for (let i = 1; i <= 5; i++) {
console.log("Counting: " + i);
}
Think of a loop like a playlist playing songs one by one until the list ends.
5. Events: Responding to User Actions
JavaScript responds to user actions like clicks, key presses, and scrolls.
document.getElementById("btn").addEventListener("click", function() {
alert("Button clicked!");
});
If a doorbell rings and you answer the door, that’s an event response.
Real-Life Uses of JavaScript
JavaScript is used in various fields, making it one of the most versatile programming languages. Here are some detailed applications:
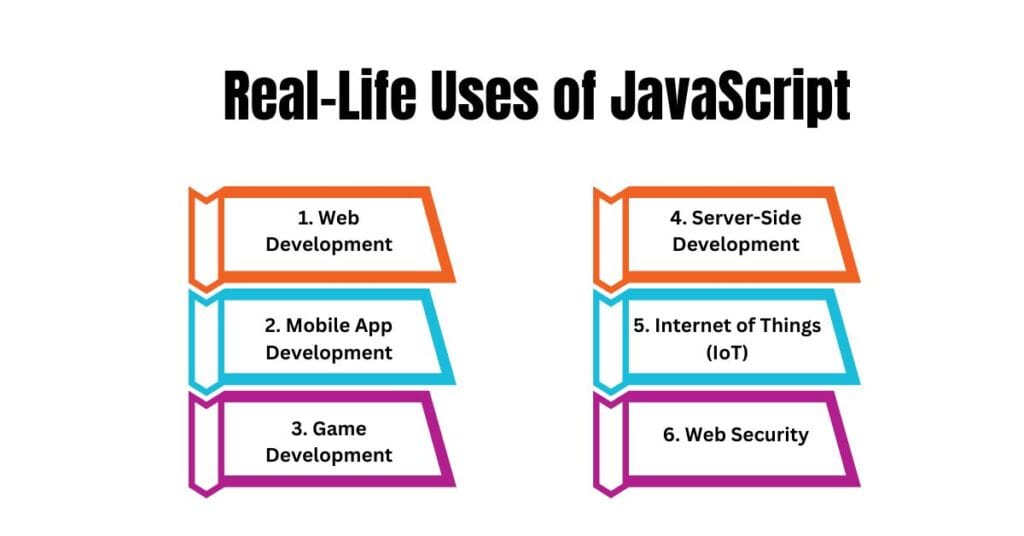
1. Web Development
JavaScript enhances websites by adding interactivity, animations, and real-time content updates. Modern frameworks like React.js, Vue.js, and Angular make web applications faster and more efficient.
2. Mobile App Development
Using frameworks like React Native and Ionic, developers can build mobile applications for both Android and iOS using JavaScript.
3. Game Development
JavaScript, along with HTML5 Canvas and WebGL, is used for creating browser-based games. Popular engines like Phaser.js help in building interactive games.
4. Server-Side Development
With Node.js, JavaScript is used for backend development, handling databases, and running APIs.
5. Artificial Intelligence and Machine Learning
JavaScript libraries like TensorFlow.js allow developers to create AI models and implement machine learning in web applications.
6. Internet of Things (IoT)
JavaScript can be used to control hardware devices like smart home automation systems using frameworks like Johnny-Five.
7. Data Visualization
Libraries such as D3.js and Chart.js enable developers to create stunning charts and graphs for data representation.
8. Web Security
JavaScript helps implement security features such as input validation, encryption, and authentication systems.
Why Learn JavaScript?
- Easy to Learn: Beginner-friendly.
- High Demand: Used by big companies like Google, Facebook, and Amazon.
- Versatile: Works on websites, mobile apps, and even servers.
- Community Support: Thousands of free tutorials and forums.
Advantages of JavaScript
JavaScript offers several benefits, making it one of the most widely used programming languages:
- Easy to Learn – JavaScript has a simple syntax, making it beginner-friendly.
- Fast Performance – JavaScript runs directly in the browser without needing additional software.
- Wide Compatibility – It works on all modern web browsers.
- Versatile – It can be used for front-end and back-end development.
- Rich User Interfaces – Enables interactive features like animations, sliders, and pop-ups.
- Large Community Support – A huge community of developers offers support and resources.
- Works with Other Technologies – JavaScript integrates well with HTML, CSS, and various backend languages.
Disadvantages of JavaScript
Despite its benefits, JavaScript has some limitations:
- Security Issues – JavaScript code is visible to users, making it vulnerable to security threats.
- Browser Differences – JavaScript may behave differently on various browsers.
- No Strict Type Checking – Unlike other languages, JavaScript does not enforce strict data types, which can lead to unexpected errors.
- Heavy on Processing – Complex JavaScript applications can slow down websites.
- Can Be Misused – Poorly written JavaScript code can make websites less efficient.
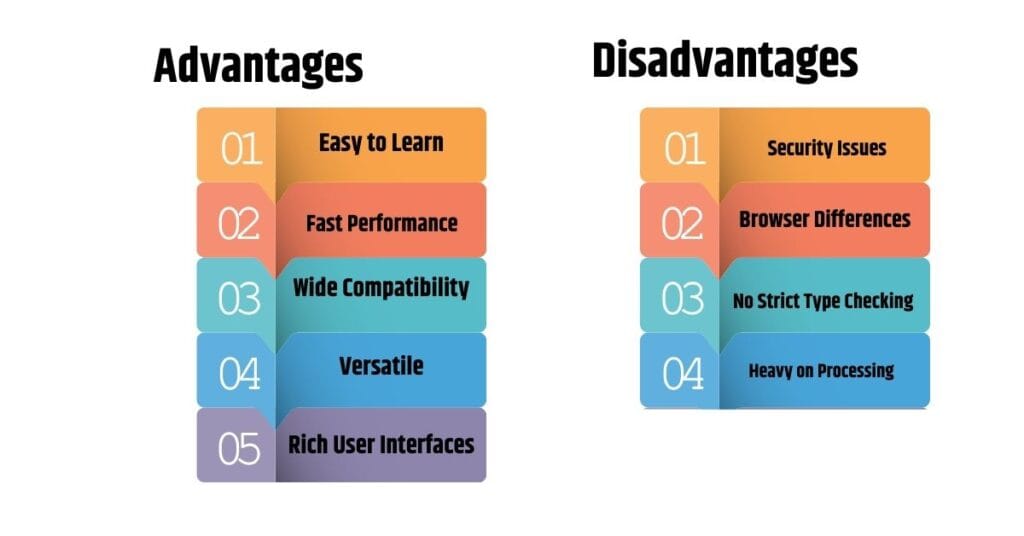
JavaScript 2025 (FAQs)
1. Is JavaScript the same as Java?
No, JavaScript and Java are completely different programming languages. JavaScript is mainly used for web development, while Java is used for applications, software development, and backend systems.
2. Can I learn JavaScript without prior programming knowledge?
Yes! JavaScript is beginner-friendly, and many resources are available to help you start from scratch.
3. Do I need to install JavaScript on my computer?
No, JavaScript runs in your browser. You can start coding using your web browser’s developer console or any code editor.
4. What can I build with JavaScript?
You can build interactive websites, games, mobile apps, and even server-side applications with JavaScript.
5. How long does it take to learn JavaScript?
It depends on your dedication. You can grasp the basics in a few weeks, but mastering JavaScript takes months of practice.
Summary
JavaScript is the backbone of interactive websites, making them dynamic and user-friendly. It allows developers to create features like form validation, animations, pop-ups, and more. Learning JavaScript is a great way to step into web development, as it is easy to learn, widely used, and has endless opportunities.
If you’re starting your journey in programming, JavaScript is the perfect place to begin. Start practicing today and explore the limitless possibilities of web development!