Introduction
JavaScript DOM 2025 – JavaScript is one of the most widely used programming languages in web development, and one of its most powerful features is the Document Object Model (DOM). The DOM allows developers to dynamically manipulate and interact with web pages.
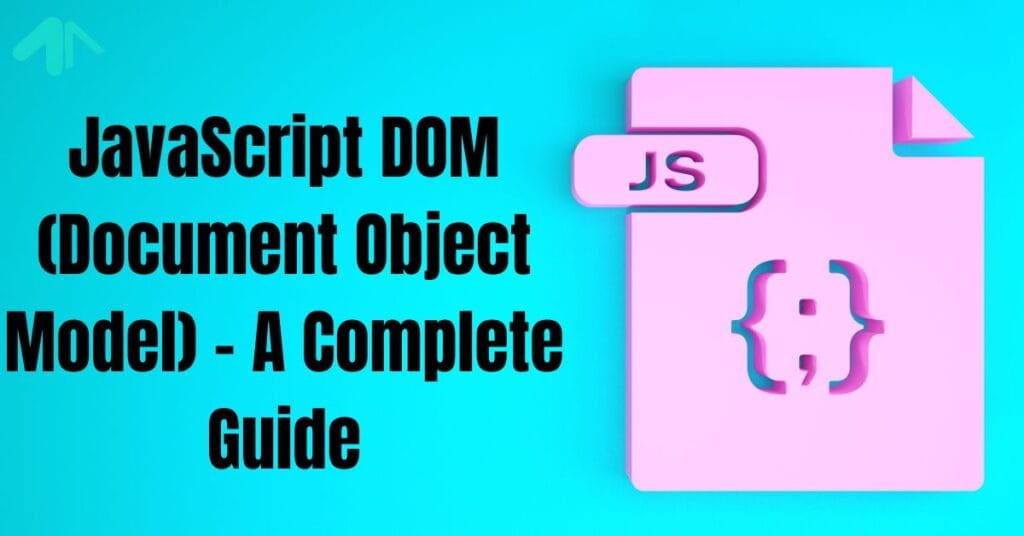
If you’ve ever seen a website where content updates without refreshing the page or buttons that change colors when clicked, that’s DOM in action!
Why is the DOM Important?
Let’s say you are reading an online article. If there is a button that says “Click me for a surprise!” and you click it, something happens—maybe a new message appears or an image changes. This magic happens because JavaScript uses the DOM to manipulate the webpage in real time.
What is the DOM?
The Document Object Model (DOM) is a representation of a web page. When a browser loads a webpage, it creates a tree-like structure of all the elements on the page. This structure is called the DOM.
Think of the DOM as a family tree where each element (HTML tag) is connected in a hierarchical order. JavaScript can access and modify this tree to dynamically change web content.
How JavaScript Interacts with the DOM
JavaScript uses the DOM to:
- Change HTML elements
- Modify CSS styles
- Respond to user actions (clicks, input, etc.)
- Add or remove elements dynamically
- Create interactive web applications
To understand how JavaScript interacts with the DOM, let’s first look at how to select elements from the webpage.
Selecting Elements in the DOM
To manipulate any part of a webpage, you first need to select it. JavaScript provides different methods to do this:
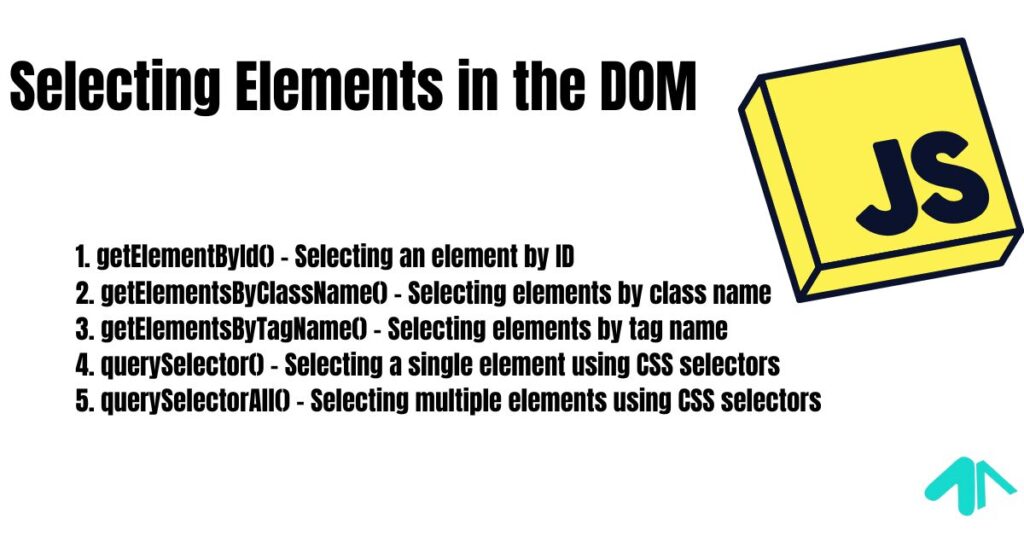
1. getElementById()
– Selecting an element by ID
let heading = document.getElementById("main-heading");
console.log(heading.innerText); // Displays the text inside the element
2. getElementsByClassName()
– Selecting elements by class name
let items = document.getElementsByClassName("list-item");
console.log(items[0].innerText); // Displays text of the first element in the list
3. getElementsByTagName()
– Selecting elements by tag name
let paragraphs = document.getElementsByTagName("p");
console.log(paragraphs.length); // Displays the number of paragraph elements
4. querySelector()
– Selecting a single element using CSS selectors
let button = document.querySelector(".btn");
button.style.backgroundColor = "blue"; // Changes button color
5. querySelectorAll()
– Selecting multiple elements using CSS selectors
let allItems = document.querySelectorAll(".list-item");
allItems.forEach(item => item.style.color = "red");
Changing HTML Content and Attributes
1. Modifying Text Content
document.getElementById("main-heading").innerText = "Welcome to JavaScript DOM!";
2. Changing HTML Attributes
document.querySelector("img").setAttribute("src", "new-image.jpg");
3. Modifying CSS Styles
document.querySelector("p").style.color = "green";
Handling Events in the DOM
Events allow interaction between users and webpages. Some common events include:
1. Click Event
document.getElementById("myButton").addEventListener("click", function() {
alert("Button Clicked!");
});
2. Mouse Hover Event
document.getElementById("myDiv").addEventListener("mouseover", function() {
this.style.backgroundColor = "yellow";
});
3. Input Event
document.getElementById("nameInput").addEventListener("input", function() {
console.log("User typed: " + this.value);
});
Adding and Removing Elements
1. Creating a New Element
let newElement = document.createElement("p");
newElement.innerText = "This is a new paragraph!";
document.body.appendChild(newElement);
2. Removing an Element
let unwantedElement = document.getElementById("oldElement");
unwantedElement.remove();
Advantages and Disadvantages of JavaScript DOM
Advantages:

- Dynamic Content – Allows real-time updates without page refresh.
- Interactivity – Enables user interaction like form validation and animations.
- Cross-Browser Support – Works in all modern browsers.
- Easy Styling – JavaScript can manipulate CSS dynamically.
Disadvantages:
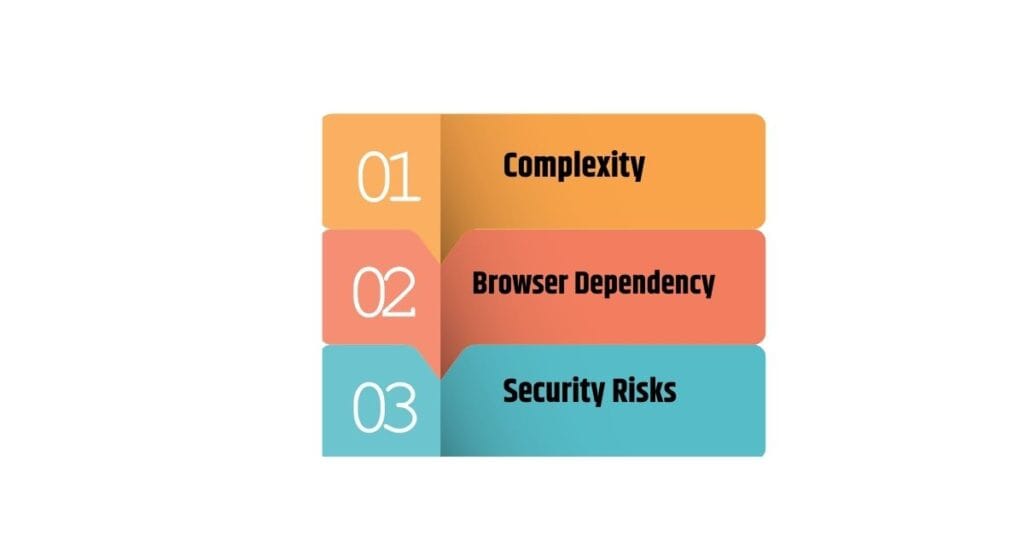
- Complexity – Large-scale DOM manipulation can slow down performance.
- Browser Dependency – Older browsers may not support all DOM features.
- Security Risks – Unsecured DOM manipulation can lead to vulnerabilities like Cross-Site Scripting (XSS).
JavaScript DOM 2025 – FAQs
1. What is the full form of DOM?
DOM stands for Document Object Model, which represents the structure of a webpage.
2. How does JavaScript modify the DOM?
JavaScript can modify the DOM using methods like getElementById()
, querySelector()
, and innerText
, among others.
3. What is the difference between innerText
and innerHTML
?
innerText
changes only the text inside an element.innerHTML
can modify both text and HTML content inside an element.
4. How do I handle events in JavaScript?
Events can be handled using addEventListener()
. For example, clicking a button can trigger an alert.
5. Why is the DOM important?
The DOM allows developers to create dynamic and interactive web applications that respond to user actions in real time.
Summary
While DOM manipulation is powerful, it has some limitations and should be optimized for performance.
- The DOM is a structured representation of a web page.
- JavaScript interacts with the DOM to modify content, styles, and attributes.
- Events allow users to interact with webpages dynamically.
- Developers can add, remove, and update elements in real time.