Recursion Function Composition and Scope in python2025
Introduction
Python is a versatile programming language that provides powerful techniques for solving problems efficiently. Among these techniques, recursion, function composition, and variable scope play a crucial role in writing clean and modular code.
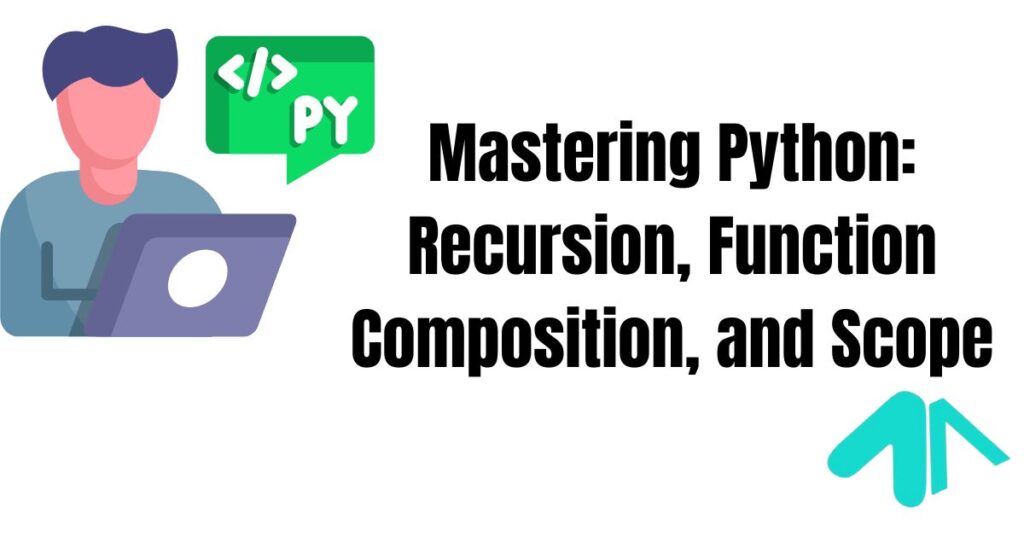
Recursion allows a function to call itself, breaking down complex problems into simpler ones. Function composition enables combining multiple functions to create a seamless workflow. Meanwhile, understanding variable scope ensures that variables are accessed and modified correctly within a program.
By mastering these concepts, programmers can improve code readability, reusability, and maintainability.
Understanding Local and Global Scope in Python
Introduction
In Python, variables have a scope, which means where they can be used in a program. There are two main types of scope:
- Local Scope – A variable declared inside a function. It can only be used inside that function.
- Global Scope – A variable declared outside all functions. It can be used anywhere in the program.
Understanding scope is important for writing clean and error-free Python programs. Let’s explore these concepts with simple examples.
Local Scope
A variable inside a function is local to that function. It cannot be used outside of it.
Example:
def greet():
message = "Hello!" # Local variable
print(message)
greet()
print(message) # Error: message is not defined outside the function
Explanation:
- The variable
message
is created insidegreet()
, so it can only be used there. - When we try to print it outside, Python gives an error.
Global Scope
A variable declared outside any function is global. It can be used inside and outside functions.
Example:
message = "Hello!" # Global variable
def greet():
print(message)
greet()
print(message) # Works fine
Explanation:
- The
message
variable is global, so it can be accessed anywhere.

Changing Global Variables Inside Functions
To modify a global variable inside a function, use the global
keyword.
Example:
count = 0 # Global variable
def increase():
global count
count += 1
increase()
print(count) # Output: 1
Explanation:
- The
global
keyword allows us to modifycount
inside the function.
The nonlocal
Keyword
If a function is inside another function, use nonlocal
to modify variables from the outer function.
Example:
def outer():
count = 0
def inner():
nonlocal count
count += 1
print(count)
inner()
outer() # Output: 1
Explanation:
nonlocal
allowsinner()
to changecount
fromouter()
.
Best Practices
- Use local variables when possible – They make the code safer and easier to debug.
- Limit global variables – Too many can make the code confusing.
- Use
global
carefully – Modifying global variables inside functions can make debugging harder. - Use constants for global values – If a global variable should not change, write it in uppercase:
MAX_USERS = 100
Common Mistakes
1. Using a local variable outside its function
def test():
value = 10 # Local variable
test()
print(value) # Error: value is not defined
Fix: Use a global variable if you need it outside the function.
2. Forgetting the global
keyword
def update():
count += 1 # Error: UnboundLocalError
Fix: Use global count
inside the function.
3. Creating a new local variable instead of modifying a global one
num = 5
def modify():
num = 10 # This creates a local variable instead of modifying the global one
modify()
print(num) # Output: 5 (unchanged)
Fix: Use global num
if modification is intended.
4. Using too many global variables
x = 10
y = 20
z = 30
def calculate():
return x + y + z
Fix: Pass variables as parameters instead.
def calculate(x, y, z):
return x + y + z
FAQs
Can I modify a global variable inside a function?
Yes, but you must use the global
keyword.
What happens if a local and global variable have the same name?
The function will use the local variable.
What is nonlocal
used for?
To modify a variable from an outer function inside a nested function.
Understanding Function Composition in Python
Introduction
Function composition is a concept in programming where multiple functions are combined to perform a task. Instead of writing long and complex functions, we create smaller, reusable functions and link them together. Python makes function composition easy and effective, helping to write cleaner and more organized code.
What is Function Composition?
Function composition means using the output of one function as the input of another. This helps in breaking a problem into smaller functions, making the code more readable and reusable.
Example:
def multiply_by_two(x):
return x * 2
def add_five(x):
return x + 5
result = add_five(multiply_by_two(3))
print(result) # Output: 11
Explanation:
multiply_by_two(3)
returns6
.add_five(6)
returns11
.- The functions are composed together to perform multiple steps.

Why Use Function Composition?
- Code Reusability – Small functions can be used in multiple places.
- Better Readability – Breaking tasks into smaller functions makes the code easier to understand.
- Easier Debugging – Smaller functions are easier to test and fix.
- Encourages Functional Programming – Reduces the dependency on variables and makes the code modular.
Function Composition with Multiple Functions
You can compose more than two functions to perform complex operations step by step.
Example:
def square(x):
return x * x
def double(x):
return x * 2
def subtract_ten(x):
return x - 10
result = subtract_ten(double(square(4)))
print(result) # Output: 22
Explanation:
square(4)
→16
double(16)
→32
subtract_ten(32)
→22
- The functions work together in a step-by-step process.
Using lambda
for Function Composition
Python allows function composition using lambda
, which makes the code even shorter.
Example:
multiply_by_three = lambda x: x * 3
subtract_two = lambda x: x - 2
composed_function = lambda x: subtract_two(multiply_by_three(x))
print(composed_function(5)) # Output: 13
Explanation:
multiply_by_three(5)
→15
subtract_two(15)
→13
- The two functions are combined into one compact function.
Function Composition with functools.reduce
If you have a list of functions, reduce
from functools
can apply them in order.
Example:
from functools import reduce
def add_three(x):
return x + 3
def multiply_four(x):
return x * 4
def divide_by_two(x):
return x / 2
functions = [add_three, multiply_four, divide_by_two]
result = reduce(lambda x, f: f(x), functions, 5)
print(result) # Output: 16.0
Explanation:
add_three(5)
→8
multiply_four(8)
→32
divide_by_two(32)
→16.0
- The
reduce
function applies each function in order.
Common Mistakes in Function Composition
1. Incorrect Order of Function Calls
def add_three(x):
return x + 3
def multiply_by_two(x):
return x * 2
print(multiply_by_two(add_three(4))) # Correct
print(add_three(multiply_by_two(4))) # May not give expected result
Fix: Always check the order in which functions should be executed.
2. Forgetting Function Returns
def step_one(x):
x + 2 # Missing return statement
def step_two(x):
return x * 3
print(step_two(step_one(5))) # Error
Fix: Always return values in each function.
3. Using Too Many Nested Functions
def a(x): return x + 1
def b(x): return x * 2
def c(x): return x - 3
def d(x): return x ** 2
def e(x): return x / 2
result = e(d(c(b(a(5)))))
print(result) # Hard to read
Fix: Use reduce
or break it into simpler steps.
FAQs – Function Composition
Can I compose more than two functions?
Yes, you can compose as many functions as needed.
What happens if I call functions in the wrong order?
You may get unexpected results or errors.
How do I simplify complex function compositions?
Use lambda
functions or functools.reduce()
to apply multiple functions in a structured way.
Understanding Recursion Composition in Python
Introduction
Recursion is a technique where a function calls itself to solve a problem. Function composition, on the other hand, involves combining multiple functions to create a new function. In Python, recursion and function composition can work together to create elegant and efficient solutions for complex problems.
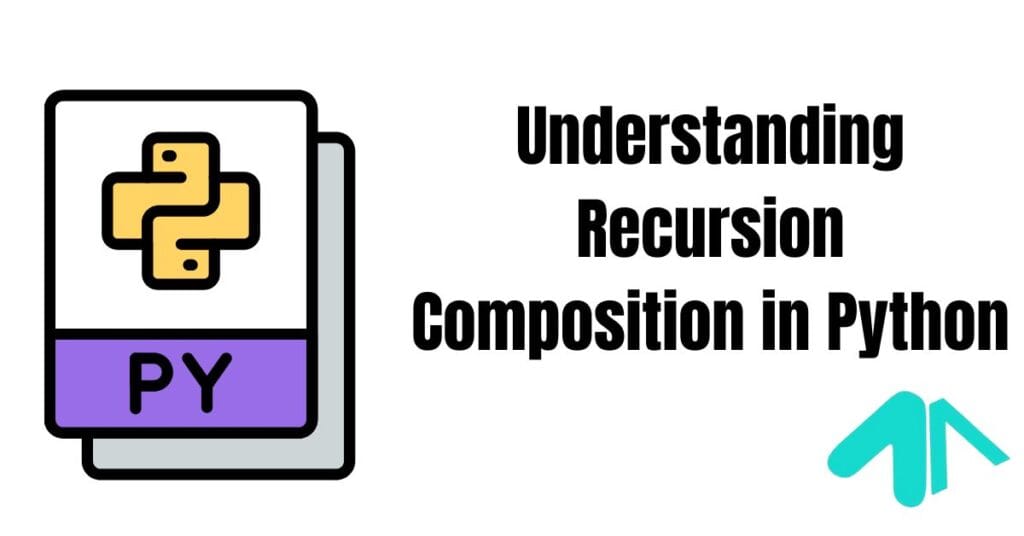
What is Recursion Composition?
Recursion composition combines recursive functions in a structured way to perform multiple operations in sequence. Instead of writing complex loops or large functions, we break down the problem into smaller functions that call each other recursively.
Example:
def multiply_by_two(n):
if n == 1:
return 2
return 2 * multiply_by_two(n - 1)
def add_five(n):
return n + 5
result = add_five(multiply_by_two(3))
print(result) # Output: 13
Explanation:
multiply_by_two(3)
→ Recursively multiplies by 2 untiln == 1
.add_five(6)
→ Adds 5 to the result of multiplication.- The functions are composed together to perform multiple steps recursively.
Why Use Recursion Composition?
- Code Reusability – Small recursive functions can be reused in multiple places.
- Better Readability – Breaking tasks into smaller recursive functions makes code easier to understand.
- Reduces Loops – Eliminates the need for traditional loops in some cases.
- Encourages Functional Programming – Helps in structuring code in a modular way.
Function Composition with Recursive Functions
You can compose recursive functions to perform complex operations step by step.
Example:
def factorial(n):
if n == 0:
return 1
return n * factorial(n - 1)
def double(n):
return n * 2
def subtract_ten(n):
return n - 10
result = subtract_ten(double(factorial(4)))
print(result) # Output: 38
Explanation:
factorial(4)
→24
double(24)
→48
subtract_ten(48)
→38
- The functions work together in a step-by-step recursive process.
Using lambda
for Recursion Composition
Python allows recursion composition using lambda
, making the code shorter.
Example:
factorial = lambda n: 1 if n == 0 else n * factorial(n - 1)
multiply_by_three = lambda x: x * 3
subtract_two = lambda x: x - 2
composed_function = lambda x: subtract_two(multiply_by_three(factorial(x)))
print(composed_function(3)) # Output: 16
Explanation:
factorial(3)
→6
multiply_by_three(6)
→18
subtract_two(18)
→16
- The functions are composed into a compact recursive function.
Function Composition with functools.reduce
If you have a list of recursive functions, reduce
from functools
can apply them in order.
Example:
from functools import reduce
def add_three(n):
return n + 3
def multiply_four(n):
return n * 4
def factorial(n):
if n == 0:
return 1
return n * factorial(n - 1)
functions = [factorial, add_three, multiply_four]
result = reduce(lambda x, f: f(x), functions, 3)
print(result) # Output: 108
Explanation:
factorial(3)
→6
add_three(6)
→9
multiply_four(9)
→36
- The
reduce
function applies each function in order.
Common Mistakes in Recursion Composition
1. Forgetting the Base Case
def recursive_function(n):
return recursive_function(n - 1) # No stopping condition
Fix: Always include a base case to stop recursion.
2. Using Too Many Nested Recursions
def a(n): return n + 1
def b(n): return n * 2
def c(n): return factorial(n - 1)
def d(n): return n ** 2
def e(n): return n / 2
result = e(d(c(b(a(5)))))
print(result) # Hard to read
Fix: Break it into smaller steps.
FAQs
What is recursion composition?
It combines recursion and function composition to solve problems step by step.
What happens if I forget the base case in recursion?
Your program will run indefinitely and may crash due to a stack overflow.
How do I simplify complex recursive function compositions?
Use lambda
functions or functools.reduce()
to apply multiple functions in a structured way.
Can I compose more than two recursive functions?
Yes, you can compose as many functions as needed.
Recursion Function Composition and Scope in python2025 -Summary
Understanding recursion, function composition, and scope in Python is essential for writing efficient and structured code. Recursion allows a function to call itself, making complex problems easier to solve by breaking them into smaller steps.
Function composition helps in creating modular programs by combining multiple functions, reducing redundancy, and improving code maintainability. Scope determines how variables are accessed and modified within a program, ensuring that functions work correctly without unintended side effects.
By applying these concepts together, developers can write cleaner, more efficient code that is easier to debug and maintain. Whether you are optimizing performance through recursion, structuring functions for better readability, or managing variable access correctly, mastering these concepts will enhance your Python programming skills significantly.
Understanding Python Parameters and Return Values: A Simple Guide