The Ultimate Guide to Using Videos in CSS 2025 – Videos are a powerful way to enhance the visual appeal of websites, providing dynamic and engaging content for users. CSS allows developers to style, position, and manipulate videos without relying heavily on JavaScript.
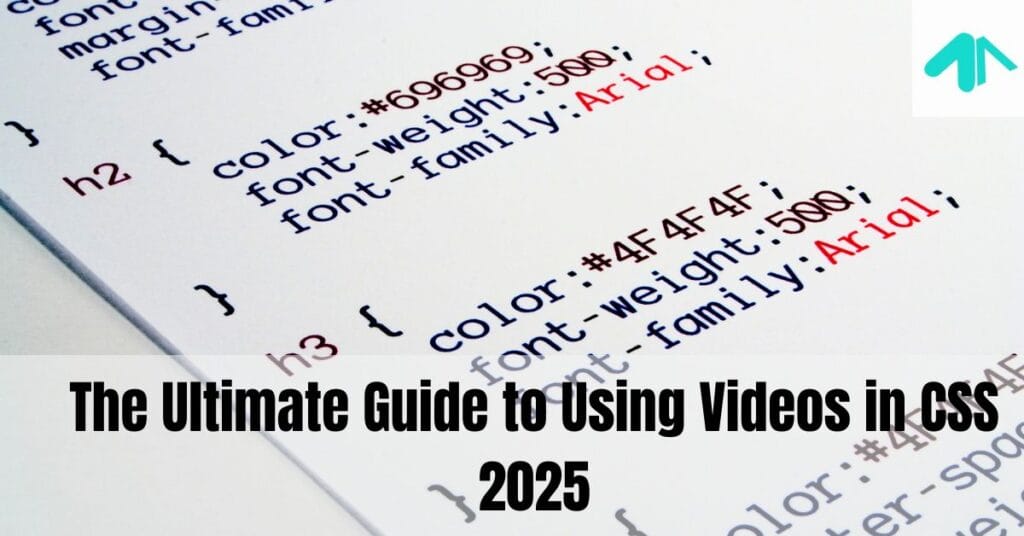
Why Use CSS for Videos?
CSS provides several benefits when working with videos, including:
- Improved Responsiveness: Ensuring videos adapt to different screen sizes.
- Enhanced User Experience: Customizing the appearance of video players.
- Reduced Dependency on JavaScript: Achieving effects like overlays, filters, and animations without scripts.
Now, let’s dive into different CSS techniques for handling videos effectively.
How to Add a Video Using HTML and CSS
Before applying CSS, we first need to add a video to our webpage. This can be done using the <video>
element in HTML.
<video class="video-style" controls>
<source src="video.mp4" type="video/mp4">
Your browser does not support the video tag.
</video>
Styling the Video with CSS
You can customize the appearance of the video with CSS:
.video-style {
width: 100%;
max-width: 800px;
display: block;
margin: auto;
border-radius: 10px;
box-shadow: 0 4px 8px rgba(0, 0, 0, 0.3);
}
This ensures that the video is centered, responsive, and visually appealing.
Making a Video Background Using CSS
A full-screen background video can create an immersive user experience. Here’s how to do it:
<div class="video-container">
<video autoplay muted loop>
<source src="background.mp4" type="video/mp4">
</video>
</div>
.video-container {
position: fixed;
top: 0;
left: 0;
width: 100%;
height: 100%;
overflow: hidden;
z-index: -1;
}
.video-container video {
width: 100%;
height: 100%;
object-fit: cover;
}
How to Make a Video Responsive with CSS
To make sure the video adapts to different screen sizes:
.video-responsive {
width: 100%;
height: auto;
}
This ensures that the video scales proportionally without distortion.
Adding an Overlay to a Video Using CSS
A semi-transparent overlay can make text more readable over a video background.
<div class="video-wrapper">
<video autoplay muted loop>
<source src="video.mp4" type="video/mp4">
</video>
<div class="overlay"></div>
</div>
.video-wrapper {
position: relative;
width: 100%;
height: 100vh;
overflow: hidden;
}
.video-wrapper video {
width: 100%;
height: 100%;
object-fit: cover;
}
.overlay {
position: absolute;
top: 0;
left: 0;
width: 100%;
height: 100%;
background: rgba(0, 0, 0, 0.5);
}
Animating a Video with CSS
CSS animations can make videos more dynamic. For example, fading in a video:
@keyframes fadeIn {
from {
opacity: 0;
}
to {
opacity: 1;
}
}
.video-style {
animation: fadeIn 2s ease-in;
}
Adding a Play Button Overlay on a Video
<div class="video-box">
<video id="video" class="video-style">
<source src="video.mp4" type="video/mp4">
</video>
<div class="play-button" onclick="document.getElementById('video').play()">▶</div>
</div>
.video-box {
position: relative;
display: inline-block;
}
.play-button {
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
font-size: 40px;
background: rgba(0, 0, 0, 0.5);
color: white;
border-radius: 50%;
padding: 20px;
cursor: pointer;
}
Videos are an essential part of modern web design. Whether you want to add a background video, style a YouTube embed, or create dynamic overlays, CSS offers many ways to enhance video elements. In this guide, we’ll cover various CSS techniques related to videos in a simple, easy-to-understand way.
How to Add a Video Background to a Widget Section in CSS
To add a video as a background in a widget section, use the <video>
element and position: absolute;
to place it behind other content.
<div class="widget-container">
<video autoplay muted loop class="background-video">
<source src="video.mp4" type="video/mp4">
</video>
<div class="content">Your Widget Content</div>
</div>
.widget-container {
position: relative;
width: 100%;
height: 300px;
overflow: hidden;
}
.background-video {
position: absolute;
top: 0;
left: 0;
width: 100%;
height: 100%;
object-fit: cover;
z-index: -1;
}
A Rotating Phone with a Video Inside Using CSS
If you want a video inside a rotating phone frame, you can use CSS animations.
<div class="phone">
<video autoplay muted loop>
<source src="video.mp4" type="video/mp4">
</video>
</div>
.phone {
width: 200px;
height: 400px;
border: 10px solid black;
border-radius: 20px;
overflow: hidden;
animation: rotate 5s infinite linear;
}
@keyframes rotate {
0% { transform: rotate(0deg); }
100% { transform: rotate(360deg); }
}
Are HTML Codes the Same as CSS for Video?
No, HTML and CSS serve different purposes:
- HTML is used to define the structure of the video element (
<video>
tag). - CSS is used to style and position the video.
Can I Display a Thumbnail of a YouTube Video Using HTML & CSS?
Yes! Use a YouTube thumbnail image as a placeholder and overlay a play button.
<a href="https://www.youtube.com/watch?v=xyz" target="_blank">
<img src="https://img.youtube.com/vi/xyz/0.jpg" alt="Thumbnail">
</a>
Replace xyz
with the actual YouTube video ID.
Can I Link a Video from CSS?
No, CSS doesn’t handle linking. You must use HTML’s <a>
tag.
Can I Put a Video in CSS Background?
Yes! Use the background
property, but it works best with video
elements instead.
body {
background: url('video.mp4') no-repeat center center fixed;
background-size: cover;
}
Can You Do Video Overlays with CSS?
Yes! Use position: absolute;
and semi-transparent backgrounds.
.overlay {
position: absolute;
top: 0;
left: 0;
width: 100%;
height: 100%;
background: rgba(0, 0, 0, 0.5);
}
Can You Loop Videos in CSS?
Looping is done using HTML’s <video loop>
attribute, not CSS.
<video loop autoplay muted>
<source src="video.mp4" type="video/mp4">
</video>
Can You Resize Videos with CSS?
Yes! Use width
and height
properties.
video {
width: 100%;
height: auto;
}
Can You Style Your YouTube Video with CSS?
Yes, but YouTube’s default player has limitations. You can embed it inside a div
and style the container.
.video-container {
position: relative;
padding-bottom: 56.25%;
height: 0;
overflow: hidden;
}
.video-container iframe {
position: absolute;
top: 0;
left: 0;
width: 100%;
height: 100%;
}
How to Add a Background Video in CSS
To add a full-page background video:
<video autoplay muted loop class="bg-video">
<source src="background.mp4" type="video/mp4">
</video>
.bg-video {
position: fixed;
top: 0;
left: 0;
width: 100%;
height: 100%;
object-fit: cover;
z-index: -1;
}
The Ultimate Guide to Using Videos in CSS 2025 – FAQs
1. Can CSS control video playback?
No, CSS cannot directly control video playback. You need JavaScript for that.
2. How can I make a video autoplay?
Use the autoplay
attribute in the <video>
tag.
3. What is object-fit: cover;
used for in videos?
It ensures that the video covers its container without stretching.
4. How do I add text over a video?
Use a <div>
inside a container and position it with CSS.
5. Can I apply CSS filters to a video?
Yes, using filter: grayscale(100%)
or similar properties.
Summary
Using CSS with videos enhances the visual appeal of your website. You can create background videos, style embedded YouTube videos, add overlays, and even animate video elements. Understanding CSS properties like position
, object-fit
, and z-index
helps you control how videos appear on your site.