Understanding 2025 Python Working
Flow of execution in Python refers to the order in which the interpreter reads and executes lines of code. It starts at the first line and continues sequentially unless the flow is changed by conditions, loops, or function calls. Knowing the flow helps in writing efficient programs and debugging errors effectively.
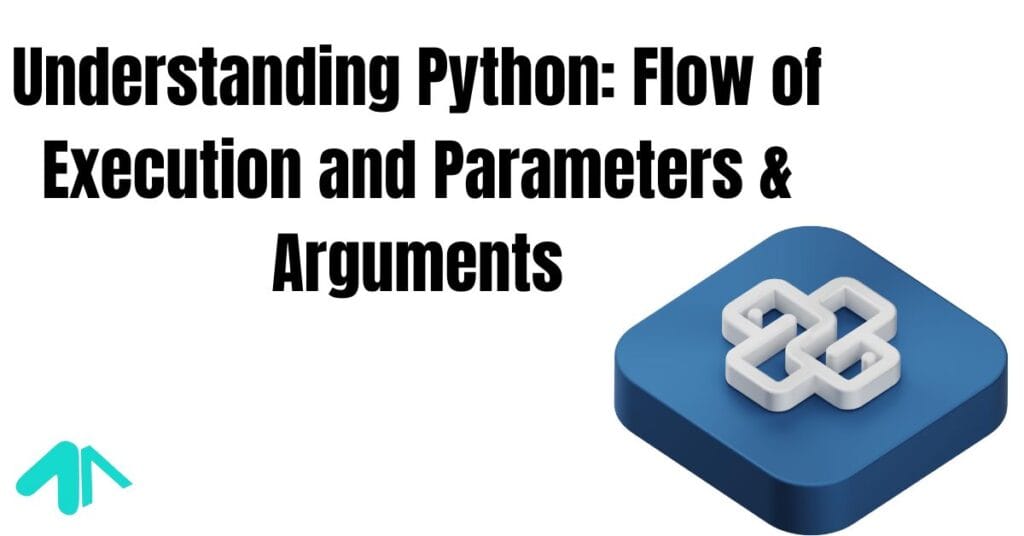
Parameters and arguments in Python are used to send information to a function. Parameters are placeholders defined in the function, while arguments are the actual values passed when calling the function. Understanding these concepts allows you to create flexible and efficient programs.
Python Flow of Execution: Understanding How Python Works
Python is a popular programming language because it is easy to read and write. To understand Python better, it is important to know how the code runs step by step. This process is called the flow of execution.
What is Flow of Execution?
Flow of execution means the order in which the Python interpreter runs the lines of code. Python reads the code from top to bottom, one line at a time, unless special instructions change the order.
When you run a Python program, the interpreter follows a systematic process. This includes starting from the first line, handling functions and loops, and finally ending the program. Knowing this flow helps you predict how your program will behave.
How Python Executes Code
Python executes code in the following stages:
- Lexical Analysis:
- Python reads the code and breaks it into small parts called tokens (e.g., keywords, variables, operators).
- Parsing:
- Python checks the structure (syntax) of the code and organizes it into a parse tree.
- Compilation:
- The code is converted into an intermediate format called bytecode.
- Execution:
- Python’s virtual machine (PVM) reads the bytecode and runs it step by step.
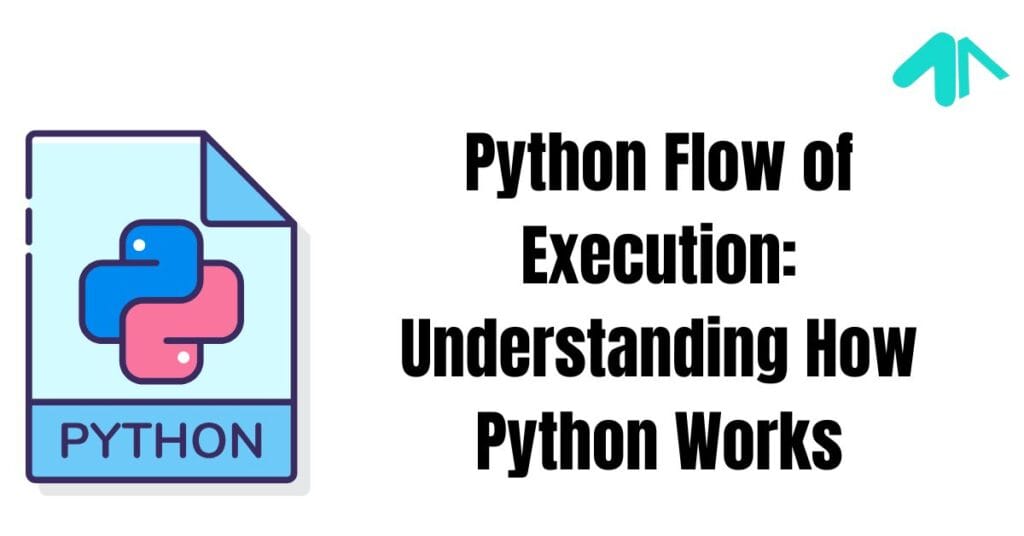
Steps in Python Flow of Execution
Here is a detailed breakdown of how Python runs a program:
- Start the Program:
- Python starts execution from the first line of the script.
- Interpret Line by Line:
- Python is an interpreted language, meaning it processes one line at a time.
- Define Functions:
- When Python encounters a function definition (
def
keyword), it stores the function for future use but does not execute it immediately.
- When Python encounters a function definition (
- Function Call:
- When a function is called, Python jumps to the function code, executes it, and then returns to where it was called.
- Conditional Statements:
- If there are
if
,elif
, orelse
statements, Python evaluates conditions and runs the corresponding block of code.
- If there are
- Loops:
- Python executes the code inside loops (
for
,while
) repeatedly until the loop condition becomes false.
- Python executes the code inside loops (
- Return Values:
- If a function has a
return
statement, it sends a value back to the caller.
- If a function has a
- End of Program:
- Once all the lines are executed, Python stops the program.
Example of Python Flow of Execution
Here is a simple Python program to show how execution flows:
# Step 1: Start
print("Program starts")
# Step 2: Define a function
def add_numbers(a, b):
print("Adding numbers")
return a + b
# Step 3: Function call
result = add_numbers(5, 10)
# Step 4: Display result
print("Result:", result)
# Step 5: End
print("Program ends")
Execution Order:
- Python prints “Program starts”.
- It defines the
add_numbers()
function but does not run it. - When
add_numbers(5, 10)
is called, Python jumps to the function. - The function prints “Adding numbers” and returns the sum (15).
- The result is displayed using the
print()
statement. - Python prints “Program ends” and the program finishes.
Flow of Execution in Different Scenarios
- Functions:
- Functions do not run until they are called.
- Python executes the function code, returns to the caller, and continues the program.
- Conditional Statements:
- Python checks conditions in the order they are written.
- Only the block with a
True
condition runs.
Example:
x = 10
if x > 5:
print("x is greater than 5")
else:
print("x is 5 or less")
- Loops:
- Loops run repeatedly until the condition is false.
Example:
count = 0
while count < 3:
print("Count:", count)
count += 1
- Recursion:
- When a function calls itself, Python stores each call in memory until the final return.
Example:
def countdown(n):
if n <= 0:
print("Done!")
else:
print(n)
countdown(n - 1)
countdown(3)
Why is Flow of Execution Important?
Understanding Python’s flow of execution helps you:
- Write Efficient Code: Plan and organize your code better.
- Debug Errors: Identify and fix mistakes quickly.
- Improve Logic: Understand how decisions and loops affect your program.
Common Mistakes in Flow of Execution
- Calling a Function Before Defining It:
- Python requires functions to be defined before they are called.
- Infinite Loops:
- Forgetting to update the loop condition causes endless execution.
Example of an infinite loop:
while True:
print("This will never stop!")
- Unreachable Code:
- Any code after a
return
statement or under aFalse
condition never runs.
- Any code after a
FAQs
Q1: What is the flow of execution in Python?
A: Flow of execution refers to the order in which Python reads and executes the lines of code, starting from the top and following specific rules for functions, loops, and conditions.
Q2: What happens if you call a function before defining it?
A: Python will raise a NameError
because it does not recognize functions until they are defined in the code.
Q3: Why are loops important in Python?
A: Loops allow Python to repeat a block of code multiple times until a condition becomes false, making tasks like iteration easier and more efficient.
Q4: Can Python execute code out of order?
A: Normally, Python runs code in order, but function calls, loops, and conditionals can change the flow by jumping to different sections.
Parameters and Arguments in Python: Understanding the Difference
When writing functions in Python, two important concepts come into play: parameters and arguments. Both are essential for passing data into a function, but they serve different purposes.
What are Parameters?
A parameter is a placeholder or variable listed inside the parentheses when defining a function. It acts like a container that holds the value you will pass when you call the function.
Think of a parameter as an empty box where you can put different things. The box stays empty until you call the function and give it a value.
Example:
# Here, 'name' is a parameter
def greet(name):
print("Hello,", name)
In this code, name
is a parameter. It will store any value you provide when you call the function.
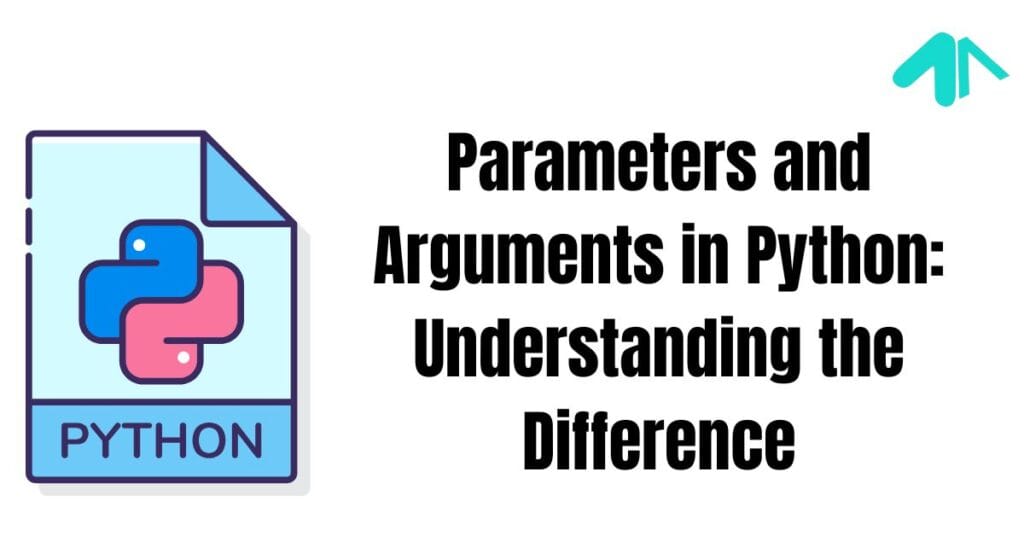
What are Arguments?
An argument is the actual value you pass to a function when you call it. This value is stored in the corresponding parameter.
When you fill the box (parameter) with an item (argument), Python uses this value during the function execution.
Example:
# Calling the function with "Alice" as an argument
greet("Alice")
Here, "Alice"
is an argument passed to the greet
function. The function prints: Hello, Alice
.
Types of Parameters and Arguments in Python
- Positional Parameters and Arguments:
- Parameters are matched with arguments based on their position.
Example:
def add(a, b):
return a + b
print(add(5, 10)) # Outputs: 15
Here, a
and b
are parameters. 5
and 10
are arguments.
- Keyword Arguments:
- You can pass arguments by specifying the parameter name.
Example:
def introduce(name, age):
print(f"My name is {name} and I am {age} years old.")
introduce(age=25, name="Bob")
Output: My name is Bob and I am 25 years old.
- Default Parameters:
- You can provide a default value for a parameter. If no argument is passed, the default value is used.
Example:
def greet(name="Guest"):
print("Hello,", name)
greet() # Outputs: Hello, Guest
greet("Alice") # Outputs: Hello, Alice
- Variable-Length Arguments:
- Sometimes, you may not know how many arguments you will receive. Python allows handling these using
*args
and**kwargs
.
- Sometimes, you may not know how many arguments you will receive. Python allows handling these using
*A. args (Non-Keyword Arguments):
def add_numbers(*numbers):
total = sum(numbers)
print("Total:", total)
add_numbers(1, 2, 3, 4) # Outputs: Total: 10
**B. kwargs (Keyword Arguments):
def print_info(**details):
for key, value in details.items():
print(f"{key}: {value}")
print_info(name="Alice", age=30, city="Pune")
Output:
name: Alice
age: 30
city: Pune
Difference Between Parameters and Arguments
Feature | Parameters | Arguments |
---|---|---|
Definition | Placeholder in function definition | Actual value passed to the function |
Usage | Defined when creating a function | Given when calling a function |
Example | def greet(name): | greet("Alice") |
Types | Positional, keyword, default, variable-length | Positional, keyword |
Why are Parameters and Arguments Important?
- Code Reusability: Functions with parameters allow you to reuse code by changing the input values.
- Flexibility: You can pass different data without rewriting the function.
- Simplifies Complex Programs: Helps break down large programs into smaller, manageable pieces.
Common Mistakes with Parameters and Arguments
- Mismatched Arguments:
- Passing fewer or extra arguments than required will cause errors.
def add(a, b):
return a + b
print(add(5)) # TypeError: missing 1 argument
- Incorrect Order:
- Mixing up the order of positional arguments can lead to wrong outputs.
def info(name, age):
print(f"Name: {name}, Age: {age}")
info(25, "Bob") # Wrong order
- Modifying Mutable Defaults:
- Avoid using mutable objects like lists as default parameters.
def add_item(item, items=[]):
items.append(item)
return items
print(add_item(1)) # [1]
print(add_item(2)) # [1, 2] (Unexpected)
To prevent this, use None
as a default and create the list inside the function.
def add_item(item, items=None):
if items is None:
items = []
items.append(item)
return items
FAQs
Q1: What is the difference between parameters and arguments in Python?
A: Parameters are variables used when defining a function. Arguments are actual values passed to the function when calling it.
Q2: Can a function have both positional and keyword arguments?
A: Yes, you can mix both types, but positional arguments must come before keyword arguments.
Q3: What happens if you pass fewer arguments than parameters?
A: Python raises a TypeError
because all required parameters must receive a value.
Q4: What is the purpose of args and kwargs?
A: args
handles multiple positional arguments, while kwargs
handles multiple keyword arguments.
Q5: Why should mutable defaults be avoided in parameters?
A: Mutable objects like lists or dictionaries can retain changes between function calls, leading to unexpected behavior.
By understanding how parameters and arguments work, you can write better and more flexible Python programs.
Understanding 2025 Python Working – Summary:
Understanding how Python processes code is essential for writing efficient and error-free programs. The flow of execution describes the order in which Python reads and runs your code. It begins at the first line and proceeds step by step unless altered by functions, loops, or conditions. Knowing the flow helps in debugging errors and predicting how your program will behave.
Parameters and arguments play a vital role in making functions flexible and reusable. Parameters are placeholders in the function definition, while arguments are actual values passed when calling a function. Python supports various argument types like positional, keyword, default, and variable-length arguments. Mastering these concepts allows you to write clean, efficient, and dynamic code while avoiding common mistakes like mismatched arguments or infinite loops.